Change TextField underline color to gradient
1,112
Though, it seems that there is not a property to change underlined color to gradient color, this effect can be achieved with Stack widget,
Here is how I tried to do it:
body: Center(
child: Container(
height: 50,
margin: EdgeInsets.all(
10.0,
),
child: Stack(
children: <Widget>[
TextField(
cursorColor: Colors.red,
decoration: InputDecoration(
hintText: " Enter your text here",
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
horizontal: 15.0,
),
border: OutlineInputBorder(
borderSide: BorderSide(
color: Colors.white,
width: 0.5,
),
borderRadius: BorderRadius.circular(
10.0,
),
),
),
),
Positioned(
bottom: -1,
child: Container(
height: 10,
width: MediaQuery.of(context).size.width - 20,
decoration: BoxDecoration(
borderRadius: BorderRadius.only(
bottomLeft: Radius.circular(10),
bottomRight: Radius.circular(10),
),
gradient: LinearGradient(
colors: [
Colors.red,
Colors.green,
],
),
),
),
),
],
),
),
),
You can modify it according to your UI.
Output:
Second Verion with no borders :
body: Center(
child: Container(
height: 50,
margin: EdgeInsets.all(
10.0,
),
child: Stack(
children: <Widget>[
TextField(
cursorColor: Colors.red,
decoration: InputDecoration(
hintText: " Enter your text here",
contentPadding: EdgeInsets.symmetric(
vertical: 15.0,
horizontal: 15.0,
),
),
),
Positioned(
bottom: 1,
child: Container(
height: 3,
width: MediaQuery.of(context).size.width - 20,
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [
Colors.red,
Colors.green,
],
),
),
),
),
],
),
),
)
Output:
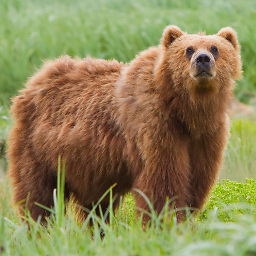
Author by
CoderUni
Updated on December 20, 2022Comments
-
CoderUni 2 days
I'm able to change the outline color of a
TextField's
color to a solid color by using the following code:TextField( decoration: InputDecoration( focusedBorder: UnderlineInputBorder( borderSide: BorderSide(color: Colors.orange), ), ), ),
However, I couldn't change its color to a gradient since it only accepts color as an input. How would I change its underline color to a linear gradient in Flutter?