Changing background color of selected cell?
Solution 1
Changing the property selectedBackgroundView is correct and the simplest way. I use the following code to change the selection color:
// set selection color
UIView *myBackView = [[UIView alloc] initWithFrame:cell.frame];
myBackView.backgroundColor = [UIColor colorWithRed:1 green:1 blue:0.75 alpha:1];
cell.selectedBackgroundView = myBackView;
[myBackView release];
Solution 2
I finally managed to get this to work in a table view with style set to Grouped.
First set the selectionStyle
property of all cells to UITableViewCellSelectionStyleNone
.
cell.selectionStyle = UITableViewCellSelectionStyleNone;
Then implement the following in your table view delegate:
static NSColor *SelectedCellBGColor = ...;
static NSColor *NotSelectedCellBGColor = ...;
- (NSIndexPath *)tableView:(UITableView *)tableView willSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
NSIndexPath *currentSelectedIndexPath = [tableView indexPathForSelectedRow];
if (currentSelectedIndexPath != nil)
{
[[tableView cellForRowAtIndexPath:currentSelectedIndexPath] setBackgroundColor:NotSelectedCellBGColor];
}
return indexPath;
}
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
[[tableView cellForRowAtIndexPath:indexPath] setBackgroundColor:SelectedCellBGColor];
}
- (void)tableView:(UITableView *)tableView willDisplayCell:(UITableViewCell *)cell forRowAtIndexPath:(NSIndexPath *)indexPath
{
if (cell.isSelected == YES)
{
[cell setBackgroundColor:SelectedCellBGColor];
}
else
{
[cell setBackgroundColor:NotSelectedCellBGColor];
}
}
Solution 3
SWIFT 4, XCODE 9, IOS 11
After some testing this WILL remove the background color when deselected or cell is tapped a second time when table view Selection is set to "Multiple Selection". Also works when table view Style is set to "Grouped".
extension ViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
if let cell = tableView.cellForRow(at: indexPath) {
cell.contentView.backgroundColor = UIColor.darkGray
}
}
}
Note: In order for this to work as you see below, your cell's Selection property can be set to anything BUT None.
How it looks with different options
Style: Plain, Selection: Single Selection
Style: Plain, Selection: Multiple Selection
Style: Grouped, Selection: Multiple Selection
Bonus - Animation
For a smoother color transition, try some animation:
extension ViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
if let cell = tableView.cellForRow(at: indexPath) {
UIView.animate(withDuration: 0.3, animations: {
cell.contentView.backgroundColor = UIColor.darkGray
})
}
}
}
Bonus - Text and Image Changing
You may notice the icon and text color also changing when cell is selected. This happens automatically when you set the UIImage and UILabel Highlighted properties
UIImage
- Supply two colored images:
- Set the Highlighted image property:
UILabel
Just supply a color for the Highlighted property:
Solution 4
// animate between regular and selected state
- (void)setSelected:(BOOL)selected animated:(BOOL)animated {
[super setSelected:selected animated:animated];
if (selected) {
self.backgroundColor = [UIColor colorWithRed:234.0f/255 green:202.0f/255 blue:255.0f/255 alpha:1.0f];
}
else {
self.backgroundColor = [UIColor clearColor];
}
}
Solution 5
-(void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = (UITableViewCell *)[tableView cellForRowAtIndexPath:indexPath];
cell.contentView.backgroundColor = [UIColor yellowColor];
}
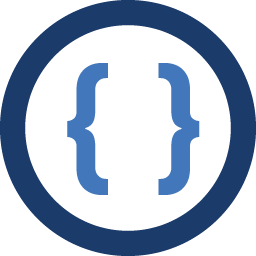
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
Does anyone know how to change the background color of a cell using UITableViewCell, for each selected cell? I created this UITableViewCell inside the code for TableView.