Changing color of label in C#, WPF, MVVM
It's getting even worse now with the updated question. Please optimize and fix your namings!
To bring this to work, here's my suggestion:
- Your DockPanelViewModel seems ok
- Create an Instance of your DockPanelViewModel and assign it to the DataContext of your View
- Bind the Foreground Property of your Label to {Binding Path=Color} (which is the Color Property of your viewModel
- Remove the "Name" of the label (you don't need that in proper MVVM
- Whenever you want to change the color of the label, just change the Color property if your viewmodel-instance (the one which you assigned to the view's DataContext)
- I have no idea what this public virtual DockPanelViewModel Label1 you recently added to the question is. For me this seems unnecessary, delete it.
Here's a working example: View:
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="*"/>
</Grid.ColumnDefinitions>
<Label Grid.Column="0" Foreground="{Binding Path=LabelColor}" Content="welcome" FontSize="20" FontWeight="Light" FontStyle="Italic"/>
<StackPanel Grid.Column="1">
<Button Content="Red" Width="75" Command="{Binding ChangeColorCommand}" CommandParameter="#FF0000"/>
<Button Content="Green" Width="75" Command="{Binding ChangeColorCommand}" CommandParameter="#00FF00" />
</StackPanel>
</Grid>
View-Code:
public MainWindow()
{
InitializeComponent();
var vm = new ViewModel();
DataContext = vm;
}
ViewModel:
public class ViewModel : INotifyPropertyChanged
{
public ICommand ChangeColorCommand { get; set; }
protected Brush _color;
public Brush LabelColor
{
get { return _color; }
set
{
_color = value;
OnPropertyChange();
}
}
public ViewModel()
{
LabelColor = Brushes.Yellow;
ChangeColorCommand = new RelayCommand((o) =>
{
LabelColor = new BrushConverter().ConvertFromString(o.ToString()) as SolidColorBrush;
});
}
public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChange([CallerMemberName] string propertyName = null)
{
var handler = PropertyChanged;
if (handler != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
}
RelayCommand is the well-known standard class found everywhere on the web.
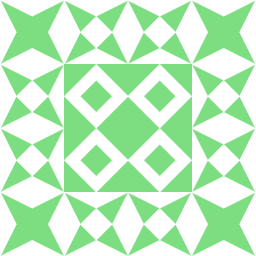
Pukaai
Updated on June 23, 2022Comments
-
Pukaai 12 months
I have made a program with several pages. It is a simple program, where I have at top area also labels at which page you currently are. For every page it is a new label defined. All labels are defined in dockpanel.xaml which is later included to mainwindow.xaml.
I like to made current page label in different color.
MY CODE:
My DockPanel.xaml for first label (others are the same only number change)
<Label Name="Label1" Foreground="{Binding Path=Label1.Color}" Content="welcome" Grid.Column="0" HorizontalAlignment="Left" FontSize="20" FontWeight="Light" FontStyle="Italic"/>
My DockPanelViewModel
public class DockPanelViewModel : ViewModelBase { #region Member fields #endregion #region Constructors /// <summary> /// The default constructor /// </summary> public DockPanelViewModel() { } #endregion #region Properties protected Brush _color; public Brush Color { get { return _color; } set { _color = value; NotifyPropertyChanged("Color"); } } #endregion }
Later definitions of ViewModel one of the page:
Label1.Color = System.Windows.Media.Brushes.Yellow;
The point is that my code dont want to change color and I dont know what is wrong :)
Please for help. Thanks!
Added .. PageViewModelBase
public virtual DockPanelViewModel Label1 { get { if (_Label1 == null) { _Label1 = new DockPanelViewModel() { //Text = "Back", Color = System.Windows.Media.Brushes.Yellow, }; } return _Label1; } set { _Label1 = value; NotifyPropertyChanged("Label1"); } }