Char type not defined
Solution 1
Char
type does not exist in VBA, you should use String
instead.
Dim myChar As String
Note that VBA is not the same as VB.NET. In VB.NET, you can use Char
.
EDIT: Following Michał Krzych's suggestion, I'd use fixed-length string for this specific purpose.
Dim myChar As String * 1
Here is an excerpt from "VBA Developer's Handbook" by Ken Getz and Mike Gilbert:
"Dynamic strings require a bit more processing effort from VBA and are, accordingly, a bit slower to use." ... "When working with a single character at a time, it makes sense to use a fixed-length string declared to contain a single character. Because you know you'll always have only a single character in the string, you'll never need to trim off excess space. You get the benefits of a fixed-length string without the extra overhead."
One caveat to this is that fixed string notation is NOT allowed in functions and subroutines;
Sub foo (char as string *1) 'not allowed
...
End Sub
So you would either need to use;
Sub foo (char as string) 'this will allow any string to pass
or
Sub foo (char as byte) 'the string would need to be converted to work
One thing to be careful of when using bytes is that there is no standard whether a byte is unsigned or not. VBA uses unsigned bytes, which is convenient in this situation.
Solution 2
I think it depends how you intend to use it:
Sub Test()
Dim strA As String, strB As Byte, strC As Integer, strD As Long
strA = "A"
strB = 65
strC = 75
strD = 90
Debug.Print Asc(strA) '65
Debug.Print Chr(strB) 'A
Debug.Print Chr(strC) 'K
Debug.Print Chr(strD) 'Z
End Sub
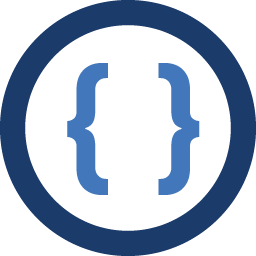
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
Dim myChar As Char
throws Compile Error: "User-defined type not defined"
What reference should I include to use Char type?