Check if a file exists with Lua
Solution 1
Try
function file_exists(name)
local f=io.open(name,"r")
if f~=nil then io.close(f) return true else return false end
end
but note that this code only tests whether the file can be opened for reading.
Solution 2
Using plain Lua, the best you can do is see if a file can be opened for read, as per LHF. This is almost always good enough. But if you want more, load the Lua POSIX library and check if posix.stat(
path)
returns non-nil
.
Solution 3
If you're using Premake and LUA version 5.3.4:
if os.isfile(path) then
...
end
Solution 4
I will quote myself from here
I use these (but I actually check for the error):
require("lfs")
-- no function checks for errors.
-- you should check for them
function isFile(name)
if type(name)~="string" then return false end
if not isDir(name) then
return os.rename(name,name) and true or false
-- note that the short evaluation is to
-- return false instead of a possible nil
end
return false
end
function isFileOrDir(name)
if type(name)~="string" then return false end
return os.rename(name, name) and true or false
end
function isDir(name)
if type(name)~="string" then return false end
local cd = lfs.currentdir()
local is = lfs.chdir(name) and true or false
lfs.chdir(cd)
return is
end
os.rename(name1, name2) will rename name1 to name2. Use the same name and nothing should change (except there is a badass error). If everything worked out good it returns true, else it returns nil and the errormessage. If you dont want to use lfs you cant differentiate between files and directories without trying to open the file (which is a bit slow but ok).
So without LuaFileSystem
-- no require("lfs")
function exists(name)
if type(name)~="string" then return false end
return os.rename(name,name) and true or false
end
function isFile(name)
if type(name)~="string" then return false end
if not exists(name) then return false end
local f = io.open(name)
if f then
f:close()
return true
end
return false
end
function isDir(name)
return (exists(name) and not isFile(name))
end
It looks shorter, but takes longer... Also open a file is a it risky
Have fun coding!
Solution 5
An answer which is windows only checks for files and folders, and also requires no additional packages. It returns true
or false
.
io.popen("if exist "..PathToFileOrFolder.." (echo 1)"):read'*l'=='1'
io.popen(...):read'*l' - executes a command in the command prompt and reads the result from the CMD stdout
if exist - CMD command to check if an object exists
(echo 1) - prints 1 to stdout of the command prompt
Related videos on Youtube
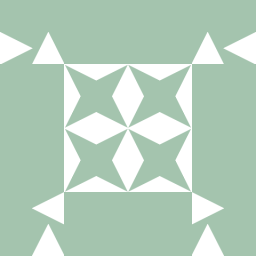
Yoni
Updated on July 05, 2022Comments
-
Yoni 6 months
How can I check if a file exists using Lua?
-
Mr. L almost 12 years@tonio - I guess more as in lua.org/pil/21.2.html
-
tonio almost 12 years@Liutauras that is even close to a real answer. I only did a quick check only on so
-
-
Lorenzo Donati support Ukraine over 9 yearsLuaFileSystem works on Windows too. Use
lfs.attributes(path,'mode')
-
Henrik Erlandsson over 8 yearsHow are errors from os.rename handled regarding renaming read-only files?
-
carpii over 7 yearsWhat are the risks in simply opening a file from lua?
-
tDwtp over 7 years@carpii If you try to open a locked file and read from it it may result in an error (you still want to know whether its a file or not). Same goes for directories (if diectory lock is supported on the host).
-
tDwtp over 7 years@HenrikErlandsson What do you mean? With 'badass error' i did not mean something you could fix by code. However, AFAIK you can use pcall to capture them. Handling might be complicated and uninformative error messages could be returned.
-
siffiejoe about 7 yearsThat would be Penlight, and it uses LuaFileSystem behind the scenes.
-
Konrad almost 4 yearsthis is not official function, it's function of premake
-
Jesse Chisholm about 3 years@Konrad AH. My mistake. premake is all I use lua for. :(
-
Konrad about 3 yearsNo problem mate
-
QuidamAzerty about 2 yearsNote: If the file is a directory, this method returns false
-
ZzZombo almost 2 yearsThis opens a briefly visible console window, so I'd advise against this idea.