Check if dictionary value of key is null
Solution 1
You can try to use ContainsKey
method to check that Name
key is exist in dictionary. Then get an actual string value by checking for null
using null-conditional ?.
operator. If the result is null
or empty string, you'll set the name to unknown
value
if (de.Properties.ContainsKey("Name"))
{
var name = de.Properties["Name"]?.ToString();
if (string.IsNullOrEmpty(name))
{
name = "unknown";
}
}
Solution 2
null
and an empty string are completely different things. null
means there's no value, while an empty string is a string, it's just empty. You can't call .ToString()
or .Length
on a null, you'd get an exception precisely because there's no value to handle that call.
You can use Dictionary.TryGetValue to check for existence and retrieve the value at the same time.
After that, if you really want to convert everything to a string, you can use the null-conditional operator ?.
to call ToString()
without throwing if name
is null. If name
is null, the entire expression name?.ToString()
evaluates to null
too.
You can then use the null-coalescing operator ??
to replace nulls with a default value :
var de= new Dictionary<string, object?>();
de["Name1"]=null;
de["Name2"]=42;
if(de.TryGetValue("Name2",out var name))
{
var valueAsString=name?.ToString() ;
parameters.Add("Name2", valueAsString ?? "unknown");
}
If you just want to replace nulls with a default value, you don't need ToString()
:
if(de.TryGetValue("Name2",out var name))
{
parameters.Add("Name2", name ?? "unknown");
}
If you want to check for null or empty, you can use String.IsNullOrEmpty :
if(de.TryGetValue("Name2",out var name))
{
var newValue=String.IsNullOrEmpty(name)
? "unknown"
: name.ToString(); //Only if we want to format to strings
parameters.Add("Name2", newValue);
}
Finally, if you want to replace existing keys in the parameters
dictionary, use
parameters["Name2]= newValue;
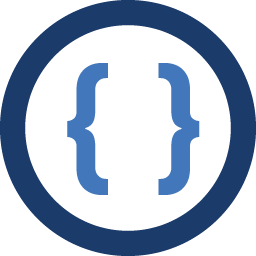
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
I try to use a Dictionary and need to Check if the Value of a Key (called Name) is empty and if so, then use some default string as Value for my Key as, something like "unknown".
I know I can check a string for example with
string.Length == 0
but my problem here is that I have an object as value and its hard for me to catch it.Thats my code:
IDictionary<string, object> parameters = new Dictionary<string, object>(); string Name = de.Properties["Name"].Value.ToString(); //Name is sometimes empty and sometimes not parameters.Add("Name", Name);
Update:
IDictionary<string, object> parameters = new Dictionary<string, object>(); string Name = de.Properties["Name"].Value.ToString(); if (parameters.TryGetValue("Name", out var Name)) { parameters.Add("Name", Name ?? "unknown"); }