Check If element exists in python selenium
A good way is to use an explicit wait. An expectation for checking that an element is present on the DOM of a page and visible. Visibility means that the element is not only displayed but also has a height and width that is greater than 0. Look at the example:
self.ui.jobs.setJobTitleEleName(titleEleName, titleText)
browser = webdriver.Chrome()
wait = WebDriverWait(browser, 5)
try:
wait.until(EC.visibility_of_element_located((By.NAME, dropdownName)))
self.ui.jobs.selectButton(dropdownName)
self.ui.sleep(4)
self.ui.jobs.selectAor(dropdownOptn)
except TimeoutException:
self.ui.jobs.selectAddAors(dropdownOptn)
self.ui.sleep(4)
self.ui.jobs.selectButton(sbmtBtnName)
The solution with if / else statement:
def provideDetails(self, titleEleName, titleText, sbmtBtnName, dropdownOptn, dropdownName):
self.ui.jobs.setJobTitleEleName(titleEleName, titleText)
browser = webdriver.Chrome()
wait = WebDriverWait(browser, 5)
elem = wait.until(EC.visibility_of_any_elements_located((By.NAME, dropdownName))) # will return a list of elements
if elem:
self.ui.jobs.selectButton(dropdownName)
self.ui.sleep(4)
self.ui.jobs.selectAor(dropdownOptn)
else:
self.ui.jobs.selectAddAors(dropdownOptn)
self.ui.sleep(4)
self.ui.jobs.selectButton(sbmtBtnName)
An expectation for checking that there is at least one element visible on a web page. locator is used to find the element returns the list of WebElements once they are located.
Imports:
from selenium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException
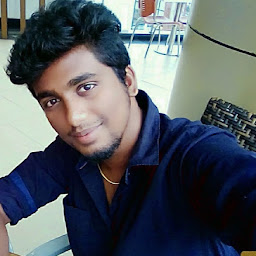
Sidharth Gokul
Updated on June 04, 2022Comments
-
Sidharth Gokul almost 2 years
Following is my python selenium code
def provideDetails(self, titleEleName, titleText, sbmtBtnName, dropdownOptn, dropdownName): self.ui.jobs.setJobTitleEleName(titleEleName, titleText) elem = self.ui.jobs.selectButton(dropdownName) if elem.is_displayed(): self.ui.jobs.selectButton(dropdownName) self.ui.sleep(4) self.ui.jobs.selectAor(dropdownOptn) else: self.ui.jobs.selectAddAors(dropdownOptn) self.ui.sleep(4) self.ui.jobs.selectButton(sbmtBtnName)
I have to check, if the 'elem' is present or not. And if it is present, 'if' condition should happen, and if not, 'else' condition should work. I tried this code. And I got this error "Attribute error: None Type object has no attribute 'is_displayed'. Any help would be appreciated. Thanks.
Also, Is there any alternative method to check if an element exists and follow with the if else commands
-
eduPeeth almost 6 yearsCan you try checking what
elem
holds before getting into the loop? -
Andersson almost 6 yearsPossible duplicate of Check if element exists python selenium
-
Sidharth Gokul almost 6 years@eduPeeth elem clicks the button.
-
murali selenium almost 6 yearsprovide code in selectButton method
-
Sidharth Gokul almost 6 years@muraliselenium def _selectSpan(self, span): """Clicks a span by text - submit, cancel, etc.""" self.ui.driver.find_element_by_xpath( "//span[contains(text(),\'{}\')]".format(span)).click() def selectButton(self, button): """Clicks a button in the Provide details page for Job, Crew, etc.""" self._selectSpan(button)
-
Sidharth Gokul almost 6 yearsor is there any alternative way to check whether an element exists ? and follow with the if else commands
-
-
Andersson almost 6 yearsIf element is found it doesn't mean that it is displayed. Do not mislead OP. You can update your code as
if elem and elem[0].is_displayed():
-
Oleksandr Makarenko almost 6 years@Andersson I'm sorry. I have rewrote my answer according to best practices. Please, check, I'm interested in your opinion.
-
Andersson almost 6 yearsOK. But now it seem that the solution doesn't meet OP requirement to use
if
/else
construction -
Oleksandr Makarenko almost 6 yearsI see. Let's leave two solutions for consideration.