Check if image exists before loading
Solution 1
The problem it does not work is getThumbnail() method will not behave as you want.
The .onload is an async call and for this case, getThumbnail(value) will always have undefined returned result;
To accomplish what you want, you can do something like:
<img src="/image/..." onerror="javascript:this.src='images/default.jpg'"/>
Solution 2
this's not working because return
is in onerror
and onload
functions, and because the request is async so function won't wait until the reuest is done.
you can achieve this using callback.
function callback(src, value){
var brick = "<div class='brick small'><a class='delete' href='#'>×</a><img src='" + src + "' /><h2><span>" + value + "</span></h2></div>";
$( ".gridly" ).append( brick );
}
function getThumbnail(name, c)
{
var image_url = "images/" + name + ".jpg";
var image = new Image();
image.onload = function()
{
c(image_url, name);
}
image.onerror = function()
{
c("images/default.jpg", name);
}
image.src = image_url;
}
$.each( cycle, function( key, value )
{
var mapIndex = key;
var mapValue = value;
getThumbnail(value, callback);
});
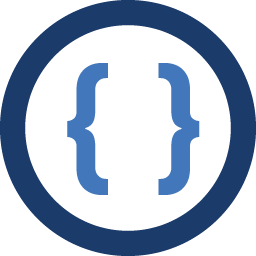
Admin
Updated on May 02, 2020Comments
-
Admin about 4 years
I've used examples from various other Stack Overflow questions and for some reason this is not working for me. I made a function to get thumbnail images and return either the actual image path or the path to the default (no thumbnail) image.
Using both the JQuery approach as well as the Javascript Image class, I get 404 errors regarding missing images in console and the found images don't error but don't show up. Can anyone perhaps point out what I am doing incorrect?
In the getThumbnail() function the "image_url" when written to console appears correct. When I am in the calling function and log the returned value, it just appears as "undefined".
JQuery:
function getThumbnail(name) { var image_url = "images/" + name + ".jpg"; $.get(image_url) .done(function() { return image_url; }) .fail(function() { return "images/default.jpg"; }) }
Javascript:
function getThumbnail(name) { var image = new Image(); image.src = image_url; image.onload = function() { return image; } image.onerror = function() { return "images/default.jpg"; } }
Calling Function:
$.each( cycle, function( key, value ) { var mapIndex = key; var mapValue = value; var brick = "<div class='brick small'><a class='delete' href='#'>×</a><img src='" + getThumbnail(value) + "' /><h2><span>" + value + "</span></h2></div>"; $( ".gridly" ).append( brick ); });
-
Admin about 9 yearsThis worked perfectly, I was not aware that those were async calls but that makes sense now. Thank you very much!
-
Admin about 9 yearsI accepted the other answer as it is much more simplistic but this makes perfect sense, thank you for the alternative approach.
-
mrid over 7 years
onerror
executes when an image has failed to load and not before loading as the OP has asked -
Cécile Fecherolle over 6 yearsPerfect! Thank you.