check if SQL row exists with PHP
60,413
Solution 1
If you would like to use PDO (PHP Data Object)
, then use the following code:
$dbh = new PDO("mysql:host=your_host_name;dbname=your_db_name", $user, $pass);
$stmt = $dbh->prepare("SELECT username from my_table where username = ':name'");
$stmt->bindParam(":name", "bob");
$stmt->execute();
if($stmt->rowCount() > 0)
{
// row exists. do whatever you want to do.
}
Solution 2
Sayem's answer has the most upvotes, but I believe it is incorrect regarding PDO.
From the PHP docs:
For most databases, PDOStatement::rowCount() does not return the number of rows affected by a SELECT statement. Instead, use PDO::query() to issue a SELECT COUNT(*) statement with the same predicates as your intended SELECT statement, then use PDOStatement::fetchColumn() to retrieve the number of rows that will be returned.
$sql = "SELECT COUNT(*) FROM fruit WHERE calories > 100";
if ($res = $conn->query($sql)) {
/* Check the number of rows that match the SELECT statement */
if ($res->fetchColumn() > 0) {
/* Issue the real SELECT statement and work with the results */
$sql = "SELECT name FROM fruit WHERE calories > 100";
foreach ($conn->query($sql) as $row) {
print "Name: " . $row['NAME'] . "\n";
}
}
/* No rows matched -- do something else */
else {
print "No rows matched the query.";
}
}
$res = null;
$conn = null;
Solution 3
another approach
$user = "bob";
$user = mysql_real_escape_string($user);
$result = mysql_query("SELECT COUNT(*) AS num_rows FROM my_table WHERE username='{$user}' LIMIT 1;");
$row = mysql_fetch_array($result);
if($row["num_rows"] > 0){
//user exists
}
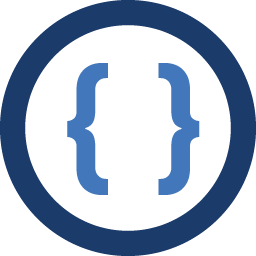
Author by
Admin
Updated on February 17, 2022Comments
-
Admin about 2 years
I'm using MySQL with PHP and I need to do something like this (pseudocode):
if (sql row exists where username='bob') { // do this stuff }