Check status if Raspberry Pi is connected to any WiFi network (Not Internet necessarily) using Python
19,726
Solution 1
Problem
iwconfig
didn't work for me, but iwgetid
did.
How do I solve this issue?
Code
import subprocess
ps = subprocess.Popen(['iwgetid'], stdout=subprocess.PIPE, stderr=subprocess.STDOUT)
try:
output = subprocess.check_output(('grep', 'ESSID'), stdin=ps.stdout)
print(output)
except subprocess.CalledProcessError:
# grep did not match any lines
print("No wireless networks connected")
Solution 2
Assuming you are running some kind of linux (like raspbian), you don't need to use Python for this, the easiest way would be:
iwconfig 2>&1 | grep ESSID
from the command line or a shell script. There will be output if you are connected to a wireless network, and no output if not. You could wrap this in subprocess
if you want to run it from Python:
import subprocess
ps = subprocess.Popen(['iwconfig'], stdout=subprocess.PIPE, stderr=subprocess.STDOUT)
try:
output = subprocess.check_output(('grep', 'ESSID'), stdin=ps.stdout)
print(output)
except subprocess.CalledProcessError:
# grep did not match any lines
print("No wireless networks connected")
Related videos on Youtube
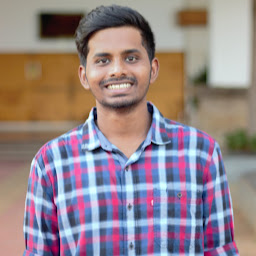
Author by
Krishnakumar M
Updated on May 26, 2022Comments
-
Krishnakumar M almost 2 years
I don't want to check if it has Internet connectivity! I just want to check if it's connected to the WiFi network (I'd have already given its SSID in the WPA_supplicant file).. This network won't have Internet access.
-
Dan Ionescu about 6 yearsDo you want to check it from the inside of the py or from the outside?
-
Krishnakumar M about 6 yearsUsing the Pi, I'll be running the Python script in the Raspberry Pi only.
-
Dan Ionescu about 6 yearsYou could consider pinging a few big websites, and consider the internet down if all are down
-
Krishnakumar M about 6 years@DanIonescu I don't want to check if it has Internet connectivity! I just want to check if it's connected to the WiFi network (I'd have already given its SSID in the WPA_supplicant file).. This network won't have Internet access. Do you get it?
-
-
Krishnakumar M about 6 yearsWorks fine! Thank you so much.
-
Austin about 3 yearsCareful with this solution. If your wifi adapter is attempting to connect to a network (even if it will be rejected due to something like an incorrect password), this command will still show the SSID as connected during the attempts.