Check synchronously if file/directory exists in Node.js
Solution 1
The answer to this question has changed over the years. The current answer is here at the top, followed by the various answers over the years in chronological order:
Current Answer
You can use fs.existsSync()
:
const fs = require("fs"); // Or `import fs from "fs";` with ESM
if (fs.existsSync(path)) {
// Do something
}
It was deprecated for several years, but no longer is. From the docs:
Note that
fs.exists()
is deprecated, butfs.existsSync()
is not. (The callback parameter tofs.exists()
accepts parameters that are inconsistent with other Node.js callbacks.fs.existsSync()
does not use a callback.)
You've specifically asked for a synchronous check, but if you can use an asynchronous check instead (usually best with I/O), use fs.promises.access
if you're using async
functions or fs.access
(since exists
is deprecated) if not:
In an async
function:
try {
await fs.promises.access("somefile");
// The check succeeded
} catch (error) {
// The check failed
}
Or with a callback:
fs.access("somefile", error => {
if (!error) {
// The check succeeded
} else {
// The check failed
}
});
Historical Answers
Here are the historical answers in chronological order:
-
Original answer from 2010
(stat
/statSync
orlstat
/lstatSync
) -
Update September 2012
(exists
/existsSync
) -
Update February 2015
(Noting impending deprecation ofexists
/existsSync
, so we're probably back tostat
/statSync
orlstat
/lstatSync
) -
Update December 2015
(There's alsofs.access(path, fs.F_OK, function(){})
/fs.accessSync(path, fs.F_OK)
, but note that if the file/directory doesn't exist, it's an error; docs forfs.stat
recommend usingfs.access
if you need to check for existence without opening) -
Update December 2016
fs.exists()
is still deprecated butfs.existsSync()
is no longer deprecated. So you can safely use it now.
Original answer from 2010:
You can use statSync
or lstatSync
(docs link), which give you an fs.Stats
object. In general, if a synchronous version of a function is available, it will have the same name as the async version with Sync
at the end. So statSync
is the synchronous version of stat
; lstatSync
is the synchronous version of lstat
, etc.
lstatSync
tells you both whether something exists, and if so, whether it's a file or a directory (or in some file systems, a symbolic link, block device, character device, etc.), e.g. if you need to know if it exists and is a directory:
var fs = require('fs');
try {
// Query the entry
stats = fs.lstatSync('/the/path');
// Is it a directory?
if (stats.isDirectory()) {
// Yes it is
}
}
catch (e) {
// ...
}
...and similarly, if it's a file, there's isFile
; if it's a block device, there's isBlockDevice
, etc., etc. Note the try/catch
; it throws an error if the entry doesn't exist at all.
If you don't care what the entry is and only want to know whether it exists, you can use path.existsSync
(or with latest, fs.existsSync
) as noted by user618408:
var path = require('path');
if (path.existsSync("/the/path")) { // or fs.existsSync
// ...
}
It doesn't require a try/catch
but gives you no information about what the thing is, just that it's there. path.existsSync
was deprecated long ago.
Side note: You've expressly asked how to check synchronously, so I've used the xyzSync
versions of the functions above. But wherever possible, with I/O, it really is best to avoid synchronous calls. Calls into the I/O subsystem take significant time from a CPU's point of view. Note how easy it is to call lstat
rather than lstatSync
:
// Is it a directory?
lstat('/the/path', function(err, stats) {
if (!err && stats.isDirectory()) {
// Yes it is
}
});
But if you need the synchronous version, it's there.
Update September 2012
The below answer from a couple of years ago is now a bit out of date. The current way is to use fs.existsSync
to do a synchronous check for file/directory existence (or of course fs.exists
for an asynchronous check), rather than the path
versions below.
Example:
var fs = require('fs');
if (fs.existsSync(path)) {
// Do something
}
// Or
fs.exists(path, function(exists) {
if (exists) {
// Do something
}
});
Update February 2015
And here we are in 2015 and the Node docs now say that fs.existsSync
(and fs.exists
) "will be deprecated". (Because the Node folks think it's dumb to check whether something exists before opening it, which it is; but that's not the only reason for checking whether something exists!)
So we're probably back to the various stat
methods... Until/unless this changes yet again, of course.
Update December 2015
Don't know how long it's been there, but there's also fs.access(path, fs.F_OK, ...)
/ fs.accessSync(path, fs.F_OK)
. And at least as of October 2016, the fs.stat
documentation recommends using fs.access
to do existence checks ("To check if a file exists without manipulating it afterwards, fs.access()
is recommended."). But note that the access not being available is considered an error, so this would probably be best if you're expecting the file to be accessible:
var fs = require('fs');
try {
fs.accessSync(path, fs.F_OK);
// Do something
} catch (e) {
// It isn't accessible
}
// Or
fs.access(path, fs.F_OK, function(err) {
if (!err) {
// Do something
} else {
// It isn't accessible
}
});
Update December 2016
You can use fs.existsSync()
:
if (fs.existsSync(path)) {
// Do something
}
It was deprecated for several years, but no longer is. From the docs:
Note that
fs.exists()
is deprecated, butfs.existsSync()
is not. (The callback parameter tofs.exists()
accepts parameters that are inconsistent with other Node.js callbacks.fs.existsSync()
does not use a callback.)
Solution 2
Looking at the source, there's a synchronous version of path.exists
- path.existsSync
. Looks like it got missed in the docs.
Update:
path.exists
and path.existsSync
are now deprecated. Please use .fs.exists
and fs.existsSync
Update 2016:
fs.exists
and been deprecated. Use fs.stat() or fs.access() instead.fs.existsSync
have also
Update 2019:
use fs.existsSync
. It's not deprecated.
https://nodejs.org/api/fs.html#fs_fs_existssync_path
Solution 3
Using the currently recommended (as of 2015) APIs (per the Node docs), this is what I do:
var fs = require('fs');
function fileExists(filePath)
{
try
{
return fs.statSync(filePath).isFile();
}
catch (err)
{
return false;
}
}
In response to the EPERM issue raised by @broadband in the comments, that brings up a good point. fileExists() is probably not a good way to think about this in many cases, because fileExists() can't really promise a boolean return. You may be able to determine definitively that the file exists or doesn't exist, but you may also get a permissions error. The permissions error doesn't necessarily imply that the file exists, because you could lack permission to the directory containing the file on which you are checking. And of course there is the chance you could encounter some other error in checking for file existence.
So my code above is really doesFileExistAndDoIHaveAccessToIt(), but your question might be doesFileNotExistAndCouldICreateIt(), which would be completely different logic (that would need to account for an EPERM error, among other things).
While the fs.existsSync answer addresses the question asked here directly, that is often not going to be what you want (you don't just want to know if "something" exists at a path, you probably care about whether the "thing" that exists is a file or a directory).
The bottom line is that if you're checking to see if a file exists, you are probably doing that because you intend to take some action based on the result, and that logic (the check and/or subsequent action) should accommodate the idea that a thing found at that path may be a file or a directory, and that you may encounter EPERM or other errors in the process of checking.
Solution 4
Another Update
Needing an answer to this question myself I looked up the node docs, seems you should not be using fs.exists, instead use fs.open and use outputted error to detect if a file does not exist:
from the docs:
fs.exists() is an anachronism and exists only for historical reasons. There should almost never be a reason to use it in your own code.
In particular, checking if a file exists before opening it is an anti-pattern that leaves you vulnerable to race conditions: another process may remove the file between the calls to fs.exists() and fs.open(). Just open the file and handle the error when it's not there.
http://nodejs.org/api/fs.html#fs_fs_exists_path_callback
Solution 5
I use below function to test if file exists. It catches also other exceptions. So in case there are rights issues e.g. chmod ugo-rwx filename
or in Windows
Right Click -> Properties -> Security -> Advanced -> Permission entries: empty list ..
function returns exception as it should. The file exists but we don't have rights to access it. It would be wrong to ignore this kinds of exceptions.
function fileExists(path) {
try {
return fs.statSync(path).isFile();
}
catch (e) {
if (e.code == 'ENOENT') { // no such file or directory. File really does not exist
console.log("File does not exist.");
return false;
}
console.log("Exception fs.statSync (" + path + "): " + e);
throw e; // something else went wrong, we don't have rights, ...
}
}
Exception output, nodejs errors documentation in case file doesn't exist:
{
[Error: ENOENT: no such file or directory, stat 'X:\\delsdfsdf.txt']
errno: -4058,
code: 'ENOENT',
syscall: 'stat',
path: 'X:\\delsdfsdf.txt'
}
Exception in case we don't have rights to the file, but exists:
{
[Error: EPERM: operation not permitted, stat 'X:\file.txt']
errno: -4048,
code: 'EPERM',
syscall: 'stat',
path: 'X:\\file.txt'
}
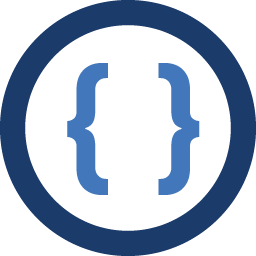
Admin
Updated on February 19, 2022Comments
-
Admin over 2 years
How can I synchronously check, using node.js, if a file or directory exists?
-
ohmantics over 13 yearsYou can link to functions in the docs. In WebKit browsers (Safari in my case), right-click and select "Inspect Element" and then find in the HTML the "id" attribute for the function you want to point to. In this case: nodejs.org/docs/v0.2.5/api.html#fs-statsync-114
-
T.J. Crowder over 13 years@ohmantics: That's strange, I thought I did exactly that (right down to using a WebKit browser to find the element ID :-) ) and it didn't work. But your link works, so clearly not. (Even the anchor is the same as what found! Weird.)
-
Paul Beusterien almost 13 yearspath.existsSync(p) is in the 0.4.10 docs nodejs.org/docs/v0.4.10/api/path.html
-
T.J. Crowder almost 13 years@Jesse:
exists
doesn't tell you whether it's a directory, it could be a file or pipe or other file system object. And surely not "grossly" overcomplicated?existsSync
would only replace thelstatSync
call, the rest of it is just to show how it would work in a loop. (Not sure why I felt that was necessary, though.) -
Hema Nandagopal almost 13 years@T.J.Crowder - Didn't mean to offend, just think that it's potentially dangerous to do this much blocking. I'd recommend at least wrapping this in
process.nextTick
calls if in a production environment. However, this is a perfectly reasonable solution if you're just scripting and it's absolutely necessary to test what kind of file it is. I just thought it was a little overkill for the OP's purposes. -
T.J. Crowder almost 13 years@Jesse: Well, I can't remember why I felt a looping example was necessary, but the OP did specifically say "synchronously", perhaps I figured the only good reason for a synchronous check was a loop...
-
Olivier Lalonde over 12 yearsActually, a more recent answer: path.existsSync is deprecated. It is now called
fs.existsSync
. -
Drew almost 12 yearspath.exists and path.existsSync have both been deprecated in favor of fs.exists and fs.existsSync
-
Ron van der Heijden over 11 yearsJust a notice:
path.existsSync
is nowfs.existsSync
-
T.J. Crowder over 11 years@Bondye: Yes. See the first paragraph of the answer. ;-)
-
Greg Hornby over 9 yearsNow the docs are saying fs.exists will be deprecated. nodejs.org/api/fs.html#fs_fs_existssync_path
-
Greg Hornby over 9 yearsis there a way to do it with openSync, rather than open
-
Melbourne2991 over 9 years@GregHornby I imagine it should work the same way with openSync
-
Ionică Bizău over 9 years
fs.exists
andfs.existsSync
will be deprecated. I created theis-there
library to replace them. @T.J.Crowder You can include it in your answer if you like. -
Ionică Bizău over 9 years
-
T.J. Crowder over 9 years@IonicăBizău: Oh for crying out loud (at them, not you). Because there's no use case for checking whether something exists unless you want to open it. sigh
-
Ionică Bizău over 9 years@T.J.Crowder Yes... I really needed to check if a file exists or not, nothing more. That's why I created
is-there
. :-) -
Josh Hansen over 9 yearsThis deprecation bugs me. Opening a file just to see if an error is thrown or not seems like a waste of resources when all that's needed is knowledge of the file's existence.
-
Matthew Dean about 9 yearsWhen I saw they marked "exists" as deprecated, definitely a head slap.
-
Petr Hurtak about 9 years"Node folks think it's dumb to check whether something exists before opening it, which it is;" Why is it dumb to check if file exists?
-
T.J. Crowder about 9 years@PetrHurtak: It isn't always (because there are lots of reasons for checking existance), but if you're going to open the file, it's best to just issue the
open
call and handle the exception or whatever if the file wasn't found. After all, the real world is chaotic: If you check first and it's there, that doesn't mean it'll still be there when you try to open it; if you check first and it isn't there, that doesn't mean it won't be there a moment later. Timing things like that seem like edge cases, but they come up all the time. So if you're going to open, no point in checking first. -
argyle almost 9 yearsAnd here I thought it was an anti-pattern to use errors for control flow: link
-
T.J. Crowder almost 9 years@jeromeyers: Indeed it is. I'd be checking the existence of the file at some relevant time, and expecting that the open call may fail. But I don't control the NodeJS API...
-
argyle almost 9 years@T.J.Crowder: So, I'll end up creating my own utility function, I think I'll call it "fsExists" ;)
-
T.J. Crowder almost 9 years@jeromeyers: You could, but Ionică has already done it for you (see comment above). :-)
-
Timothy C. Quinn over 8 years@Dan, thanks. I removed the truncated text. I cannot recall what the note was. If it comes me I will add notes.
-
Dan Dascalescu over 8 yearsNp. I'm deleting my comment.
-
bob over 8 yearsNice, I added || isDirectory() to make it a file/folder checker. var stats = fs.statSync(filePath);return stats.isFile() || stats.isDirectory();
-
Ionică Bizău over 8 yearsI wrote a little library to replace the old
exists
function:is-there
-
Admin over 8 yearsnodejs.org/api/fs.html#fs_fs_existssync_pathStability: 0 - Deprecated: Use fs.statSync() or fs.accessSync() instead.
-
aurora over 8 yearsIt's an anti-pattern to use errors for control flow and it leads to much more ulgy code, in my opinion. So they are deprecating a function, that others have to re-implement in own libraries? WTF ...
-
basickarl over 8 yearsUpvote for keeping the answer updated, superb. Wish more people would do this.
-
broadband over 8 yearsIf program doesn't have rights to access the file it still returns false even though file exists i.e. remove all rigts from file chmod ugo-rwx file.txt or in Windows Right Click ... Exception message: Exception fs.statSync (./f.txt): Error: EPERM: operation not permitted, stat 'X:\f.txt'. So this case isn't covered by upper code.
-
1mike12 almost 8 yearsReally like this, it's one of the few answers that's up to date since node has deprecated the last 37 ways of doing this
-
expelledboy over 7 yearsWow, JS is retarded sometimes. So sure, 97% of the time you will be using the file, but not having a simple
file.exists()
util for the 3% and instead forcing us to wrap this in a try catch? Get real... Bitch of the day. -
Eugene over 7 yearsLOL. Im checking this post like couple years, and every time something new )))
-
Jamie Hutber almost 7 yearsNow the only question I have is, how do you get the path? By this I mean, I don't know if the file isn't there or i'm just getting the path wrong :O
-
jgmjgm over 6 yearsBah, you beat me to it. I could have saved some time if I had read this.
-
stackdave over 6 years@T.J.Crowder please can you include the node minimal version for your last update answer?
-
T.J. Crowder over 6 years@stackdave: It's at the top of the answer:
existsSync
. Or am I misunderstanding? -
Kunok over 6 yearscurrenct docs (version ~9) only labeled
fs.exists
as deprecated whilefs.existsSync
is not! -
nirazul over 6 yearsThe only reason I don't upvote this is the counter: 1337
-
T.J. Crowder over 6 years@Nirazul: ROFL
-
HaulinOats over 6 yearsThese updates were a rollercoaster of emotion. Thank you for being so thorough.
-
vdegenne about 6 yearsthe OP wants a synchronous solution
-
jgmjgm about 6 yearsThe original question does not specify that. I am also demonstrating how to do things unambiguously. Many answers might induce bugs due to lack of clarity. People often want to program things so it appears syncronous but don't necessarily want synchronous execution. statSync is not the same as the code I've demonstrated. Either accounts of what's actually desired are ambiguous, so you're only imposing your personal interpretations. If you find an answer you don't understand it might be better to simply ask in the comments or PM to work out what edits are needed.
-
jgmjgm about 6 yearsIf you want you can also steal my code sample, name it appropriately, put it on github, add it to npm and then the answer will only be one line/link :D.
-
jgmjgm about 6 yearsThe code is short for sake of example but you're welcome to submit an edit suggestion to include && !isFile or a check for symlinks, etc (again though the question never explicitly states even that is what they want). As I have already pointed out my answer satisfies one interpretation of the question and does not do the same thing your one line proposal does.
-
Kip about 6 yearsI can't remember ever seeing something get un-deprecated.
-
bluenote10 over 5 yearsYour 2016 update is just the opposite of the 2016 update in the accepted answer... Why?
-
T.J. Crowder about 5 years@mikemaccana - Ah yes, good thing to add now that the API is stable!
-
Francesco Casula about 5 years
fs.exists()
is deprecated, butfs.existsSync()
is not, check the docs, you're reporting false information nodejs.org/api/fs.html#fs_fs_existssync_path -
pery mimon almost 5 yearsyou should update your code to
function asyncFileExists(path) { //F_OK checks if file is visible, is default does no need to be specified. return new Promise(function (res, rej) { fs.access( path, fs.constants.F_OK, function (err) { err ? rej(err) : res(true); }, ); }); }
-
unknown over 3 yearsVery useful! Thanks
-
TamusJRoyce over 3 years"It's an anti-pattern to use errors for control flow" - normally this would be the case. But filesystem errors are handled using atomic operations coming from the Southbridge / CPU. Since many threads can access files, using errors means file-access is thread-safe and you get consistent results. Since this is OS level, this applies to all languages including 16mhz 286 cpu from the 80's.
-
PSXGamerPro1 about 3 yearsOr just open the file in creation mode and lock it from being used by other processes (which prevents it from being deleted until the process that locked it deletes it).