Check/ Uncheck All checkboxes - AngularJS ng-repeat
16,393
Solution 1
If you are using Angular 1.3+ you can use getterSetter
from ng-model-options
to solve this and avoid manually keeping track of the allSelected
state
HTML:
<input type="checkbox" ng-model="allSelected" ng-model-options="{getterSetter: true}"/>
JS:
var getAllSelected = function () {
var selectedItems = $scope.Items.filter(function (item) {
return item.Selected;
});
return selectedItems.length === $scope.Items.length;
}
var setAllSelected = function (value) {
angular.forEach($scope.Items, function (item) {
item.Selected = value;
});
}
$scope.allSelected = function (value) {
if (value !== undefined) {
return setAllSelected(value);
} else {
return getAllSelected();
}
}
Solution 2
You can use this function to check if all records are checked whenever a checkbox changes:
$scope.checkStatus= function() {
var checkCount = 0;
angular.forEach($scope.Items, function(item) {
if(item.Selected) checkCount++;
});
$scope.selectedAll = ( checkCount === $scope.Items.length);
};
The view code:
<input type="checkbox" ng-model="item.Selected" ng-change="checkStatus();"/>
http://jsfiddle.net/TKVH6/840/
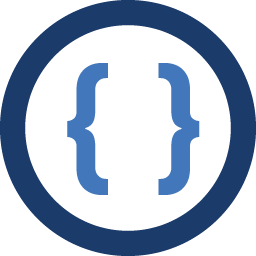
Author by
Ramya
Updated on June 04, 2022Comments
-
Ramya almost 2 years
I have a structure which looks like this: http://jsfiddle.net/deeptechtons/TKVH6/
<div> <ul ng-controller="checkboxController"> <li>Check All <input type="checkbox" ng-model="selectedAll" ng-click="checkAll()" /> </li> <li ng-repeat="item in Items"> <label>{{item.Name}} <input type="checkbox" ng-model="item.Selected" /> </label> </li> </ul> </div> angular.module("CheckAllModule", []) .controller("checkboxController", function checkboxController($scope) { $scope.Items = [{ Name: "Item one" }, { Name: "Item two" }, { Name: "Item three" }]; $scope.checkAll = function () { if ($scope.selectedAll) { $scope.selectedAll = true; } else { $scope.selectedAll = false; } angular.forEach($scope.Items, function (item) { item.Selected = $scope.selectedAll; }); }; });
When check all is selected or deselected, all other checkboxes are selected. But when "Check All" is selected, and I deselect one of the items, I want "Check All" to be deselected.
Thanks in advance!
(PS: Thanks to deeptechtons for the JSFiddle)
-
rob almost 9 yearsSorry I meant to edit mine but I accidentally edited yours. Did I revert it to the right jsFiddle link?