Checking if device is iPad
15,476
Solution 1
You can use
if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiomPad)
Solution 2
In Swift you can use:
if UIDevice.current.userInterfaceIdiom == .pad {
//do stuff
}
Solution 3
you must cheack the condition wheather device is iphone/ipad
if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiomPad)
{
//do ur ipad logic
}else
{
//do ur iphone logic
}
Solution 4
If you are using swift, use this
if (UI_USER_INTERFACE_IDIOM() == UIUserInterfaceIdiom.Pad)
{
// device is ipad
}
else
{
// device is iPhone
}
Solution 5
/* The UI_USER_INTERFACE_IDIOM() function is provided for use when deploying to a version of the iOS less than 3.2. If the earliest version of iPhone/iOS that you will be deploying for is 3.2 or greater, you may use -[UIDevice userInterfaceIdiom] directly. */
Better use UIDevice.current.userInterfaceIdiom
unless you are supporting super old iOS.
Possible cases are as below:
public enum UIUserInterfaceIdiom : Int {
case unspecified
@available(iOS 3.2, *)
case phone // iPhone and iPod touch style UI
@available(iOS 3.2, *)
case pad // iPad style UI
@available(iOS 9.0, *)
case tv // Apple TV style UI
@available(iOS 9.0, *)
case carPlay // CarPlay style UI
}
Related videos on Youtube
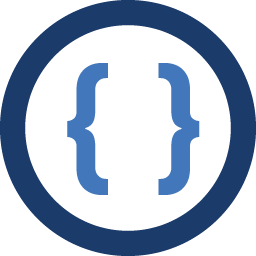
Author by
Admin
Updated on July 02, 2022Comments
-
Admin almost 2 years
What is wrong with this code? I want to be able to check if the current device the user is using is an iPad but it keeps giving me errors.
if (UIUserInterfaceIdiom == UIUserInterfaceIdiomPad) { //do stuff }
-
epascarello over 11 yearsYou probably want to tag this with more than just ipad.
-
Daniel Monteiro over 11 yearsMaybe you're calling it too late? Are you loading your XIB manually? I remember doing this and succeeding. But I guess there are better solutions.
-
-
magma over 11 yearsNote that an UIUserInterfaceIdiomPhone value does not necessarily mean that you're on an iPhone device - rather, that the interface idiom matches the iPhone one. You might be on an iPad, running an iPhone-only app in 1x/2x mode.
-
rmaddy over 11 yearsActually it's UI_USER_INTERFACE_IDIOM().
-
Makleesh over 11 yearsThat's an interesting point magma thanks for bringing it to my attention. I had no idea that was the case