Checking if string is only letters and spaces
The function can be written more simpler and correctly if to use standard C functions isalpha
and isblank
declared in header <ctype.h>
For example
#include <ctype.h>
//...
int checkString( const char s[] )
{
unsigned char c;
while ( ( c = *s ) && ( isalpha( c ) || isblank( c ) ) ) ++s;
return *s == '\0';
}
If you want to check whether a string contains white spaces then instead of function isblank
you should use function isspace
.
Take into account that it is not a good idea to use statement continue
in such simple loops. It is better to rewrite the loop without the continue
statement.
And instead of function scanf
it is better to use function fgets
if you want to enter a sentence The function allows to enter several words as one string until the Enter will be pressed.
For example
fgets( str1, sizeof( str1 ), stdin );
Take into account that the function includes the new line character. So after entering a string you should remove this character. For example
size_t n = strlen( str1 );
if ( n != 0 && str1[n-1] == '\n' ) str1[n-1] = '\0';
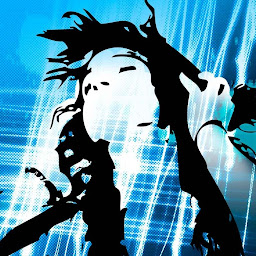
n4tri0s
Updated on June 24, 2022Comments
-
n4tri0s almost 2 years
I wrote this simple code to check if a string is letters and spaces only
#include<stdio.h> #include<conio.h> #include<math.h> #include<stdlib.h> #include<string.h> #define N 100 int checkString(char str1[]); void main() { char str1[N]; scanf("%s", str1); printf("%d",checkString(str1)); getch(); } int checkString(char str1[]) { int i, x=0, p; p=strlen(str1); for (i = 0; i < p ; i++) { if ((str1[i] >= 'a' && str1[i] <= 'z') || (str1[i] >= 'A' && str1[i] <= 'Z') || (str1[i] == ' ')) { continue; } else{ return 0; } } return 1; }
This works fine when I type something like :
hello asds //returns 1 hello1010 sasd // return 0
but if I type anything after space it returns
1
, like this :hello 1220 //returns 1 blabla 11sdws // returns 1
Can someone please tell me why?
-
WhozCraig almost 9 yearsYou missed the point of the question. That numbers are entered is precisely the reason the OP desires function to return 0 for a string such as "hello 123". The problem is the method of reading the string, which stops upon the first whitespace.
-
user35443 almost 9 years@WhozCraig buh... Yes, I have definitely missed it then.