chrome extension - reading from serial port
First the example does not work properly. Try this instead:
var connectionId;
$(document).ready(function() {
chrome.serial.getDevices(function(devices) {
for (var i = 0; i < devices.length; i++) {
$('select#portList').append('<option value="' + devices[i].path + '">' + devices[i].path + '</option>');
}
});
// ui hook
$('button#open').click(function() {
var clicks = $(this).data('clicks');
if (!clicks) {
var port = $('select#portList').val();
chrome.serial.connect(port, {bitrate: 9600}, function(info) {
connectionId = info.connectionId;
$("button#open").html("Close Port");
console.log('Connection opened with id: ' + connectionId + ', Bitrate: ' + info.bitrate);
});
} else {
chrome.serial.disconnect(connectionId, function(result) {
$("button#open").html("Open Port");
console.log('Connection with id: ' + connectionId + ' closed');
});
}
$(this).data("clicks", !clicks);
});
});
Now as for the actual reading of the input from the serial connection it will work but converting the ArrayBuffer to a string is a bit harder than expected.
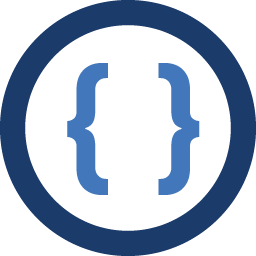
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
This is my first attempt at developing with a chrome app or extension. I have a GPS receiver on the USB port which is emulated as a serial device.
Running this code
var onGetDevices = function(ports) { for (var i=0; i<ports.length; i++) { // show me some output console.log(ports[i].path); // Connect to the serial port /dev/ttyUSB0 chrome.serial.connect(ports[i].path, {bitrate: 9600}, onConnect); } } chrome.serial.getDevices(onGetDevices);
gets me "/dev/ttyUSB0" in the console, so it appears to be finding the device.
How do I then connect to the device? I've included the serial.connect line above, with the following functions:
var onConnect = function(connectionInfo) { // The serial port has been opened. Save its id to use later. _this.connectionId = connectionInfo.connectionId; // Do whatever you need to do with the opened port. chrome.serial.onReceive.addListener(onReceiveCallback); } var stringReceived = ''; var onReceiveCallback = function(info) { if (info.connectionId == expectedConnectionId && info.data) { var str = convertArrayBufferToString(info.data); if (str.charAt(str.length-1) === '\n') { stringReceived += str.substring(0, str.length-1); onLineReceived(stringReceived); stringReceived = ''; } else { stringReceived += str; } } };
but I get the following error:
Error in response to serial.connect: ReferenceError: _this is not defined at Object.onGetDevices [as callback]
I'm not sure exactly what I'm doing right or wrong here so any pointers appreciated.
-
Admin over 9 yearsawesome, thanks Joe, this really set me up on the right track. cant believe the chrome tutorial doesnt have proper working code..!
-
Joe Kasavage over 9 yearsNot a problem. Took me a little to figure it out myself.
-
Admin over 9 yearsJoe - im having issues connecting to the port now. Its weird, just doesnt seem to want to play ball. Did you get something up and running your end?
-
Joe Kasavage over 9 yearsYes I used this exact code to read from the serial device, barcode scanner in this instance. I am assuming it is failing to open even when you set the bitrate?
-
Admin over 9 yearsyes, i've just downloaded a packaged app and it cant connect either, so must be my hardware.its a USB GPS device which emulates as a serial port. It works fine on linux using gpsmon, so must be down to being a usb device and not natively serial?
-
Joe Kasavage over 9 yearsThat may be the case. Try thr chrome.usb lib and see if that helps.
-
Admin over 9 yearsLet us continue this discussion in chat.