chrome.webRequest.onBeforeRequest.addListener Cannot read property 'onBeforeRequest' of undefined
Solution 1
The comments above don't make sense to me. There is a background script above and it seems to have appropriate permissions... Some extra comments that might help...
You need to add a background page in the manifest file and the appropriate permissions in the manifest so the background page can access the webRequest APIs. See this example: chrome.webRequest not working?
As Mihai mentioned, if you need to have the content script perform the action, check this page out: https://developer.chrome.com/extensions/messaging
Add this to your content script (you can change greeting to action and hello to which action the background script should perform):
chrome.runtime.sendMessage({greeting: "hello"}, function(response) {
console.log(response.farewell);
});
Add this to your background page (you can do if statements and peform different actions based on the message):
chrome.runtime.onMessage.addListener(
function(request, sender, sendResponse) {
console.log(sender.tab ?
"from a content script:" + sender.tab.url :
"from the extension");
if (request.greeting == "hello")
sendResponse({farewell: "goodbye"});
});
Solution 2
I just ran into a similar issue, except the problem for me was unrelated to permissions and it was in fact a Webpack issue.
My background script was accidentally being bundled with my content script. Background js was exporting certain constants that content script was using, causing methods restricted to background file (such as chrome.webRequest
) to be called as part of content script, hence the cannot read property of undefined…
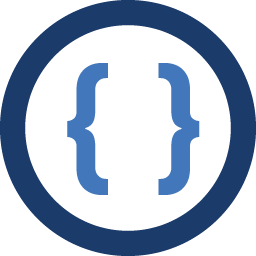
Admin
Updated on July 20, 2022Comments
-
Admin almost 2 years
I'm trying to build my own chrome extension and I'm trying to add an event handler using
onBeforeRequest
.My
manifest.json
:{ "manifest_version": 2, "name": "My extension", "description": "some descrpition", "version": "1.0", "permissions": [ "activeTab", "tabs", "webRequest", "webNavigation", "management", "http://*/*", "https://*/*" ], "background": { "scripts": [ "js/jquery-2.1.4.min.js", "js/background.js" ], "persistent": true }, "browser_action": { "default_icon": "imgs/img.png", "default_title": "extension" }, "icons" : { "64" : "imgs/vergrootglas.png" } }
My
background.js
:function callback(param1,param2,param3){ alert(param1); alert(param2); alert(param3); } //alert("test"); chrome.webRequest.onBeforeRequest.addListener(callback);
I got this loaded into my chrome. But everytime I get this message in my console:
Uncaught TypeError: Cannot read property 'onBeforeRequest' of undefined
I can't figure out what I'm oding wrong, I've found this: https://developer.chrome.com/extensions/webRequest
But the example of the code seems to be quite the same as what I do. What am I missing here?