Class and accessor methods Java
Solution 1
An accessor method is used to return the value of a private or protected field. It follows a naming scheme prefixing the word "get" to the start of the method name. For example let's add accessor methods for name:
class Player{
protected name
//Accessor for name
public String getName()
{
return this.name;
}
}
you can access the value of protected name through the object such as:
Player ball = new Player()
System.out.println(ball.getName())
A mutator method is used to set a value of a private field. It follows a naming scheme prefixing the word "set" to the start of the method name. For example, let's add mutator fields for name:
//Mutator for name
public void setName(String name)
{
this.name= name;
}
now we can set the players name using: ball.setName('David');
Solution 2
Your instance variables are age and name. Your setter methods are void and set your passed arguments to the corresponding variable. Your getters are not void and return the appropriate variables.
Try this and come back with questions.
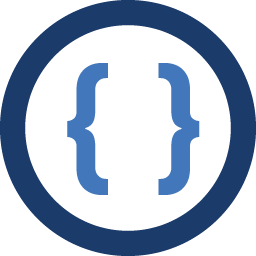
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I don't understand accessor methods and I'm stuck with creating setAge, getAge and getName.
This is the question:
Add three accessor methods,
setAge
,getAge
andgetName
. These methods should set and get the values of the corresponding instance variables.public class Player { protected int age; protected String name; public Player(String namArg) { namArg = name; age = 15; } }