class std::vector<...> has no member named
15,261
Solution 1
You have an array of vectors for some reason.
vector<animal> masses[2*c];
should probably be
vector<animal> masses(2*c);
Solution 2
Here are the ways to defining and initializing vector:
vector<T> v;//empty
vector<T> v1(v2);//v1 is a copy of v2
vector<T> v1 = v2;
vector<T> v1{a, b, c};//list initialize
vector<T> v1 = {a, b, c};
vector<T> v(b, e);// v is a copy of the elements in the range denoted by iterators b and e
vector<T> v(n);//v has n value-initialized elements
vector<T> v(n, t);// v has n elements with value t
So, your defining doesn't do what you want,
vector<animal> masses[2*c]; //masses is an array of vector<animal>
when you say masses[i] which is a vector not animal, has no member named ‘p’
You should write:
vector<animal> masses(2*c);
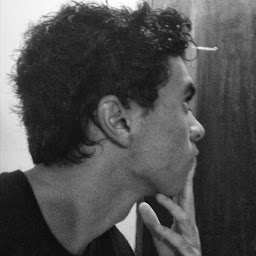
Author by
hrss
Updated on June 04, 2022Comments
-
hrss almost 2 years
I'm pretty new to C++, and I'm trying to use a vector of structs, but I keep getting "class std::vector’ has no member named ‘p’" when I try to compile my code. This is it, by the way:
#include <vector> #include <cstdlib> #include <stdio.h> #include <algorithm> using namespace std; struct animal { int p; int v; }; bool cmpp (animal a, animal b) { return (a.p < b.p); } bool cmpv (animal a, animal b) { return (a.v < b.v); } bool cmpc (pair<animal, animal> a, pair<animal, animal> b) { return (a.first.p < b.first.p); } int main(){ int c, s; while(scanf("%d %d", &c, &s) != EOF){ vector<animal> masses[2*c]; vector<pair<animal, animal> > chamber; for (int i = 0; i < 2*c-s; i++){ masses[i].v = 0; masses[i].p = -1; } for (int i = 2*c-s; i < 2*c; i++){ masses[i].v << cin; masses[i].p << i - 2*c-s; } sort(masses.begin(), masses.end(), cmpv); for(int i = 0; i < c; i++){ if(chamber[i].p < masses[2*c-i] && chamber[i].p != -1) chamber[i] = pair<animal, animal> (masses[i], masses[2*c-i]); else chamber[i] = pair<animal, animal> (masses[2*c-i], masses[i]); } sort(chamber.begin(), chamber.end(), cmpc); for(int i = 0; i < c; i++){ printf("%d %d", chamber[i].first.v, chamber[i].second.v) } } return 0; }
There might be other errors in the code, but I just wanna know the vector thing for now. P.S.: This is my first question here :) I knew this day would come!