Classpath problems:running ant java task with Maven Antrun plugin
Solution 1
I think I've hit on the solution to this one after being frustrated for some time : inspired by this thread
The antrun plugin is correctly constructing classpath references, but not passing them through to the external build file when you invoke the ant
task.
So the solution is to explicitly pass in any classpath references you want to access using the <reference>
element.
<!-- antrun plugin execution -->
<plugin>
<artifactId>maven-antrun-plugin</artifactId>
<version>1.7</version>
<executions>
<execution>
<id>build</id>
<phase>compile</phase>
<goals>
<goal>run</goal>
</goals>
<configuration>
<target>
<ant antfile="${basedir}/build.xml">
<!-- This is the important bit -->
<reference torefid="maven.compile.classpath" refid="maven.compile.classpath"/>
</ant>
</target>
</configuration>
</execution>
</executions>
</plugin>
And consume them as normal in your ant build task
<!-- External ant build referencing classpath -->
<java classname="net.nhs.cfh.ebook.Main" fork="true" failonerror="true">
<arg value="-b"/>
<arg value="${dist.dir}"/>
<arg value="-o"/>
<arg value="${xml.dir}/treeindex"/>
<arg value="tree.xml"/>
<jvmarg value="-Dstrategy=treeParser"/>
<!-- reference to the passed-in classpath reference -->
<classpath refid="maven.compile.classpath"/>
</java>
Solution 2
in maven:
<plugin>
<artifactId>maven-antrun-plugin</artifactId>
....
<property name="compile_classpath" refid="maven.compile.classpath"/>
....
in Ant using pathelement instead of path refid
<path id="classpath">
<pathelement path="${compile_classpath}"/>
</path>
then it work
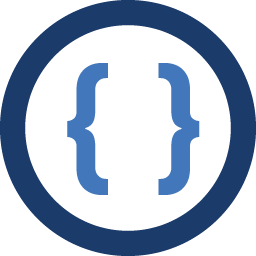
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
When running ant task from maven ant run plugin I can set maven classpath as an ant property. However when I try to run
<ant:java
task setting this exact classpath I get the error that the reference can not be find. As if the whole classpath is interpreted as one jar. Is there a way to somehow set this classpath to ant java task?(from maven)
<plugin> <artifactId>maven-antrun-plugin</artifactId> .... <property name="compile_classpath" refid="maven.compile.classpath"/> ....
(from ant) ...
<path id="classpath"> <path refid="${compile_classpath}"/> </path> ... <java classname="..." classpathref="classpath"> ... </java>
The version of maven ant run plugin is 1.7
If this can not be done is there some way in ant to iterate this classpath string (location of jar files with ';' separator) and set the values of jar location as '