Clear TEXTAREA value after click (onFocus)
Solution 1
It's better to put instructions into a label tag, position it over the textarea, and then hide it or show it if the textarea is focused. The reason for this is because your textearea will be populated with instructions and these might get sent if they are inside a form.
But, solve your problem, all you have to do is:
onfocus="if(this.value== "your initial text"){this.value=''}; return false;"
Solution 2
I think it's a good habit to seperate the logic from the content. So, using jQuery (we all use jQuery, do we?) you could do:
$(document).ready(function() {
var _bCleared = false;
$('form textarea').each(function()
{
var _oSelf = $(this);
_oSelf.data('bCleared', false);
_oSelf.click(function()
{
if (!_oSelf.data('bCleared'))
{
_oSelf.val('');
_oSelf.data('bCleared', true);
}
});
});
});
This will iterate over all the textareas on the site and store a boolean value about their clear state in a seperate data binding. The onclick event is also handled separately for each textarea, so you can have multiple textarea / textarea clearings on a single page and no need for Javascript splattering your html.
Solution 3
this will help
function clearTA {
if(this.value != "type here") {
this.value = '';
return false;
}
}
and onfocus html attribute
...onfocus="clearTA()"..
Solution 4
<script type="text/javascript">
function clearOnInitialFocus ( fieldName ) {
var clearedOnce = false;
document.getElementById( fieldName ).onfocus = (function () {
if (clearedOnce == false) {
this.value = '';
clearedOnce = true;
}
})
}
window.onload = function() { clearOnInitialFocus('myfield');
</script>
Source: http://snipplr.com/view/2206/clear-form-field-on-first-focus/
Solution 5
I know this is late, but to anyone else having this problem I believe the simplest answer is
onfocus="this.value=''; this.onfocus='';"
should do exactly what you want without having to store/check any variables.
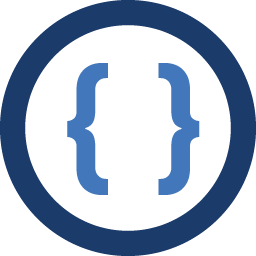
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm currently using..
onfocus="this.value=''; return false;"
to clear the value within my textarea for leaving comments. However, when you click to enter a comment and type a few things, if you were to click outside the box to maybe read something else on the page and then return to your comment by clicking within the textarea box again, it clears your current input.
Is there anyway to clear the textarea on the initial click just to clear the default value and not the rest of the clicks for the rest of the browser page's session?