clearTimeout() not working
22,149
Solution 1
The clearTimeout
is working, but you are immediately re-setting it in the same method. So it starts up again right away. You need to conditionally check for whether to start it:
slideshow: function(sw) {
var timer;
if (sw == "off") {
clearTimeout(timer);
} else {
timer = setTimeout(function() { Gallery.next(); }, 1000);
}
}
Solution 2
The way you structured your code is more complex than it needs to be and has a few bugs. I'd recommend restructuring it like this:
var Gallery = {
timer: null,
next: function() {
/* do stuff */
},
close: function() {
if (Gallery.timer) {
clearInterval(Gallery.timer);
Gallery.timer = null;
}
},
slideshow: function() {
Gallery.close();
Gallery.timer = setInterval(function() {
Gallery.next();
}, 1000);
}
}
You'd start the slideshow with Gallery.slideshow();
and stop it with Gallery.close();
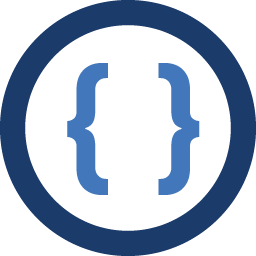
Author by
Admin
Updated on July 23, 2020Comments
-
Admin almost 4 years
In the following code the clearTimeout() function doesn't seem to clear the timer. Please note: I've stripped the code down a bit to show the relevent parts. Any ideas?
var Gallery = { next: function() { // does stuff }, close: function() { Gallery.slideshow("off"); }, slideshow: function(sw) { if (sw == "off") {clearTimeout(timer);} var timer = setTimeout(function() {Gallery.next();Gallery.slideshow();}, 1000); }, };
FULL CODE:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Gallery</title> <meta name="description" content=" "> <meta name="author" content=" "> <link rel="stylesheet" href="scripts/css.css"> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.min.js"></script> <!--[if lt IE 9]> <script src="http://html5shiv.googlecode.com/svn/trunk/html5.js"></script> <![endif]--> <script> var Gallery = { // PARAMETERS FOR CUSTOMISATION gallery_type: "overlay", // overlay(default), inpage, slideshow transition_speed: 1000, // milliseconds outer_opacity: 0.7, // 0 to 1 (option available with overlay) outer_colour: "#000000", // hex value image_size: "800px", show_captions: true, // true, false overlay_slideshow: true, // true, false (option available with overlay) slideshow_interval: 5000, // milliseconds slideshow_controls: false, // true, false fullscreen: false, // true, false (option available with inpage) thumbs: [], images: [], captions: [], current_image_id: null, timer: null, init: function() { // CREATE ARRAYS var gallery_div = document.getElementById("gallery"); gallery_thumbs = gallery_div.getElementsByTagName("img"); for (var i=0; i < gallery_thumbs.length; i++) { Gallery.thumbs[i] = gallery_thumbs[i]; Gallery.thumbs[i].rel = [i]; } for (var i=0; i < gallery_thumbs.length; i++) { Gallery.captions[i] = Gallery.thumbs[i].alt; } // CREATE OVERLAYS var body_tag = document.getElementsByTagName("body"); var outer_overlay = document.createElement("div"); outer_overlay.id = "outer"; outer_overlay.style.backgroundColor = Gallery.outer_colour; var window_height = $(document).height(); outer_overlay.style.height = window_height +'px'; body_tag[0].appendChild(outer_overlay); var inner_overlay = document.createElement("div"); inner_overlay.id = "inner"; body_tag[0].appendChild(inner_overlay); var close_button = document.createElement("img"); close_button.src="images/icon-close.png"; close_button.id = "gallery-close"; inner_overlay.appendChild(close_button); var next_button = document.createElement("img"); next_button.src="images/icon-next.png"; next_button.id = "gallery-next"; inner_overlay.appendChild(next_button); var prev_button = document.createElement("img"); prev_button.src="images/icon-prev.png"; prev_button.id = "gallery-prev"; inner_overlay.appendChild(prev_button); var caption_div = document.createElement("div"); caption_div.id = "gallery-caption"; inner_overlay.appendChild(caption_div); $("#gallery-caption").fadeTo(0, 0); if (Gallery.show_captions == true) { inner_overlay.onmouseover = function() {$("#gallery-caption").stop().fadeTo(Gallery.transition_speed/2, 0.5);}; inner_overlay.onmouseout = function() {$("#gallery-caption").stop().fadeTo(Gallery.transition_speed, 0);}; } // CREATE IMAGES for (var i=0; i < Gallery.thumbs.length; i++) { var new_image = document.createElement("img"); new_image.src = Gallery.thumbs[i].parentNode.href; new_image.id= "gallery-img" + (i + 1); inner_overlay.appendChild(new_image); Gallery.images[i] = new_image; $(Gallery.images[i]).fadeTo(0, 0); } // EVENT HANDLERS FOR OPEN AND CLOSE for (var i=0; i < Gallery.thumbs.length; i++) { Gallery.thumbs[i].onclick = Gallery.open; } var outer_overlay = document.getElementById("outer"); outer_overlay.onclick = Gallery.close; close_button.onclick = Gallery.close; next_button.onclick = Gallery.next; prev_button.onclick = Gallery.previous; }, open: function() { Gallery.current_image_id = this.rel; var current = Gallery.images[Gallery.current_image_id]; var caption_div = document.getElementById("gallery-caption"); caption_div.innerHTML = Gallery.captions[Gallery.current_image_id]; var inner_overlay = document.getElementById("inner"); inner_overlay.style.height = current.height + "px"; // CENTER BUTTONS & OVERLAY var next_button = document.getElementById("gallery-next"); next_button.style.top = (current.height / 2) -15 + "px"; var prev_button = document.getElementById("gallery-prev"); prev_button.style.top = (current.height / 2) -15 + "px"; $("#inner").css("top", (($(window).height()-$("#inner").height())/2)); $("#inner").css("left", (($(window).width()-$("#inner").width())/2)); $(current).fadeTo(0, 1); $("#outer").fadeTo(0, Gallery.outer_opacity); $("#inner").fadeTo(0, 0); $("#inner").fadeTo(Gallery.transition_speed, 1); if (Gallery.overlay_slideshow == true) { Gallery.slideshow(); } return false; }, close: function() { if (Gallery.timer) { clearInterval(Gallery.timer); Gallery.timer = null; } else { $("#outer").fadeTo(0, 0); $("#inner").fadeTo(0, 0); $("#outer").css("display", "none"); $("#inner").css("display", "none"); var current = Gallery.images[Gallery.current_image_id]; current.style.display = "none"; } }, next: function() { var next_id = Number(Gallery.current_image_id) + 1; if (next_id == Gallery.images.length) {next_id = 0;} var next = Gallery.images[next_id]; var current = Gallery.images[Gallery.current_image_id]; var inner_overlay = document.getElementById("inner"); inner_overlay.style.height = next.height + "px"; // CENTER BUTTONS & OVERLAY var next_button = document.getElementById("gallery-next"); next_button.style.top = (next.height / 2) -15 + "px"; var prev_button = document.getElementById("gallery-prev"); prev_button.style.top = (next.height / 2) -15 + "px"; $("#inner").css("top", (($(window).height()-$("#inner").height())/2)); $("#inner").css("left", (($(window).width()-$("#inner").width())/2)); var caption_div = document.getElementById("gallery-caption"); caption_div.innerHTML = Gallery.captions[next_id]; $(current).fadeTo(Gallery.transition_speed, 0); $(next).fadeTo(0, 0); $(next).fadeTo(Gallery.transition_speed, 1); Gallery.current_image_id = next_id; }, previous: function() { var prev_id = Number(Gallery.current_image_id) - 1; if (prev_id == -1) {prev_id = Gallery.images.length -1;} var prev = Gallery.images[prev_id]; var current = Gallery.images[Gallery.current_image_id]; var inner_overlay = document.getElementById("inner"); inner_overlay.style.height = prev.height + "px"; // CENTER BUTTONS & OVERLAY var next_button = document.getElementById("gallery-next"); next_button.style.top = (prev.height / 2) -15 + "px"; var prev_button = document.getElementById("gallery-prev"); prev_button.style.top = (prev.height / 2) -15 + "px"; $("#inner").css("top", (($(window).height()-$("#inner").height())/2)); $("#inner").css("left", (($(window).width()-$("#inner").width())/2)); var caption_div = document.getElementById("gallery-caption"); caption_div.innerHTML = Gallery.captions[prev_id]; $(current).fadeTo(Gallery.transition_speed, 0); $(prev).fadeTo(0, 0); $(prev).fadeTo(Gallery.transition_speed, 1); Gallery.current_image_id = prev_id; }, slideshow: function() { Gallery.close(); Gallery.timer = setInterval(function() { Gallery.next(); }, 1000); }, fullscreen: function() { } }; // START SCRIPTS Start = function() { Gallery.init(); }; window.onload = Start; </script> </head> <body> <div id="gallery"> <a href="images/argentina-river.jpg"><img src="images/thumbs/argentina-river-thumb.jpg" alt="Argentina River"></a> <a href="images/monte-bre.jpg"><img src="images/thumbs/monte-bre-thumb.jpg" alt="Monte Bre"></a> <a href="images/romania.jpg"><img src="images/thumbs/romania-thumb.jpg" alt="Romania"></a> <a href="images/norway.jpg"><img src="images/thumbs/norway-thumb.jpg" alt="Norway"></a> <a href="images/cloudy-skies.jpg"><img src="images/thumbs/cloudy-skies-thumb.jpg" alt="Cloudy Skies"></a> <a href="images/field.jpg"><img src="images/thumbs/field-thumb.jpg" alt="Field"></a> <a href="images/poland.jpg"><img src="images/thumbs/poland-thumb.jpg" alt="Poland"></a> <a href="images/coconut-trees.jpg"><img src="images/thumbs/coconut-trees-thumb.jpg" alt="Coconut Trees"></a> <a href="images/volcanic-land.jpg"><img src="images/thumbs/volcanic-land-thumb.jpg" alt="Volcanic Land"></a> <a href="images/tokyo-city.jpg"><img src="images/thumbs/tokyo-city-thumb.jpg" alt="Tokyo City"></a> </div> </body> </html>