COBOL: File status 39 error
The FILE STATUS 39 means that there is a difference between what you have told COBOL about the file and what COBOL has discovered whilst attempting to OPEN it.
For the file you have defined in COBOL, the data must be exactly 99 bytes long. If you have 99 bytes per record, followed by one (or more) delimiters, then you'd need to extend the length of INREC to 100 or 101 bytes.
You may want to see if your compiler (from the error message it seems to be CA-Realia) supports LINE SEQUENTIAL
files. This particular file-type understands delimited records, and the delimiters will be stripped before being presented to your program.
The simplest file-processing program in COBOL follows this outline:
OPEN files
READ input until end
process input, WRITE output
CLOSE files
That read-loop will typically be one of two forms:
PERFORM until some-flag-shows-end-of-file
READ input-file
AT END
make end-of-file-flag show "end of file"
NOT AT END
do some processing
END-READ
END-PERFORM
READ input-file ("priming read")
PERFORM until FILE STATUS field shows end-of-file
do some processing
READ input-file
END-PERFORM
The second is, to my mind, much simpler and less error-prone. It requires that the FILE STATUS is used on the SELECT for the file. This really should always be done for all files so that you can check after each IO that the IO didn't behave unexpectedly. Separate FILE STATUS field for each file.
Here's your program re-arranged. At the moment, it only reads one record (or none if end-of-file is returned immediately) but that is what you coded.
PROCEDURE DIVISION.
PERFORM INIT-RTN
PERFORM PROCESS-FILE
PERFORM END-RTN
PERFORM FINISH-RTN
STOP RUN
.
INIT-RTN.
OPEN INPUT INFILE
check file status
OPEN OUTPUT OUTFILE
check file status
PERFORM HEADING-RTN
.
PROCESS-FILE.
READ INFILE
check file status
.
END-RTN.
DISPLAY 'EMPTY FILE' LINE 3 COLUMN 20
.
HEADING-RTN.
WRITE OUTREC FROM HEAD-1 AFTER PAGE
check file status
WRITE OUTREC FROM HEAD-2 AFTER 1
check file status
WRITE OUTREC FROM HEAD-3 AFTER 1
check file status
WRITE OUTREC FROM HEAD-4 AFTER 3
check file status
WRITE OUTREC FROM SUB-1 AFTER 2
check file status
WRITE OUTREC FROM SUB-2 AFTER 1
check file status
.
PROCESS-RTN.
DISPLAY SCRE
.
FINISH-RTN.
CLOSE INFILE
check file status
CLOSE OUTFILE
check file status
DISPLAY 'TAPOS NA' LINE 6 COLUMN 20
.
So, check on FILE STATUS, LINE SEQUENTIAL, change your file (or program definition of it). Build from there.
You may think the FILE STATUS checking of the report lines will look large and ugly. You can do them like this, instead:
MOVE HEAD-1 TO OUTREC
PERFORM WRITE-OUTREC-AFTER-PAGE (other neater ways to do it later)
Where WRITE-OUTREC has the WRITE and the test of the FILE STATUS field.
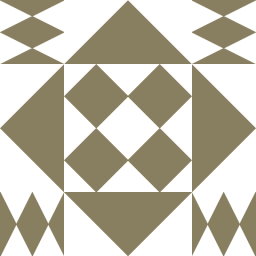
xzbxxzxzmn
Updated on June 04, 2022Comments
-
xzbxxzxzmn about 1 year
here is my cobol code. i have the testinput.txt in my cobol folder. I compiled it and it has no errors but when i try to run it,
there's a message that says RCL0002: File status 39 on < unopened-file > Error detected at offset 0046 in segment 00 of program TEST
IDENTIFICATION DIVISION. PROGRAM-ID. transactionIN. ENVIRONMENT DIVISION. CONFIGURATION SECTION. SOURCE-COMPUTER. IBM-PC. OBJECT-COMPUTER. IBM-PC. INPUT-OUTPUT SECTION. FILE-CONTROL. SELECT INFILE ASSIGN TO 'testinput.txt'. SELECT OUTFILE ASSIGN TO 'testoutput'. DATA DIVISION. FILE SECTION. FD INFILE LABEL RECORD IS STANDARD DATA RECORD IS INREC. 01 INREC. 02 AC PIC X(99). FD OUTFILE LABEL RECORD IS OMITTED DATA RECORD IS OUTREC. 01 OUTREC. 02 FILLER PIC X(80). WORKING-STORAGE SECTION. 01 HEAD-1. 02 FILLER PIC X(32) VALUE SPACES. 02 FILLER PIC X(16) VALUE 'China Trust Bank'. 02 FILLER PIC X(32) VALUE SPACES. 01 HEAD-2. 02 FILLER PIC X(34) VALUE SPACES. 02 FILLER PIC X(13) VALUE 'Makati Avenue'. 02 FILLER PIC X(33) VALUE SPACES. 01 HEAD-3. 02 FILLER PIC X(35) VALUE SPACES. 02 FILLER PIC X(12) VALUE 'Makati City'. 02 FILLER PIC X(34) VALUE SPACES. 01 HEAD-4. 02 FILLER PIC X(33) VALUE SPACES. 02 FILLER PIC X(14) VALUE 'Account Report'. 02 FILLER PIC X(33) VALUE SPACES. 01 SUB-1. 02 FILLER PIC X(20) VALUE SPACES. 02 FILLER PIC X(7) VALUE 'Account'. 02 FILLER PIC X(10) VALUE SPACES. 02 FILLER PIC X(7) VALUE 'Account'. 02 FILLER PIC X(9) VALUE SPACES. 02 FILLER PIC X(7) VALUE 'Balance'. 02 FILLER PIC X(20) VALUE SPACES. 01 SUB-2. 02 FILLER PIC X(20) VALUE SPACES. 02 FILLER PIC X(6) VALUE 'Number'. 02 FILLER PIC X(12) VALUE SPACES. 02 FILLER PIC X(4) VALUE 'Name'. 02 FILLER PIC X(10) VALUE SPACES. 02 FILLER PIC X(18) VALUE SPACES. SCREEN SECTION. 01 SCRE. 02 BLANK SCREEN. PROCEDURE DIVISION. MAIN-RTN. PERFORM INIT-RTN THRU INIT-RTN-END. PERFORM FINISH-RTN. STOP RUN. INIT-RTN. OPEN INPUT INFILE, OUTPUT OUTFILE. READ INFILE AT END PERFORM END-RTN GO TO INIT-RTN-END. PERFORM HEADING-RTN. INIT-RTN-END. END-RTN. DISPLAY 'EMPTY FILE' LINE 3 COLUMN 20. HEADING-RTN. WRITE OUTREC FROM HEAD-1 AFTER PAGE. WRITE OUTREC FROM HEAD-2 AFTER 1. WRITE OUTREC FROM HEAD-3 AFTER 1. WRITE OUTREC FROM HEAD-4 AFTER 3. WRITE OUTREC FROM SUB-1 AFTER 2. WRITE OUTREC FROM SUB-2 AFTER 1. PROCESS-RTN. DISPLAY SCRE. FINISH-RTN. CLOSE INFILE, OUTFILE. DISPLAY 'TAPOS NA' LINE 6 COLUMN 20.