CodeIgniter From Post Data Not Going through
Solution 1
You can add this form attribute:
enctype="multipart/form-data;charset=utf-8"
Instead of:
enctype="multipart/form-data"
You can see this link.
Solution 2
You need fix your do_upload
, like this..
$this->upload->do_upload('name-of-input-file-element-of-your-form')
For example, in your view code, you have:
<input type="file" name="userfile" size="20" />
So, your do_upload
line, should be like this:
$this->upload->do_upload('userfile')
Saludos!
Solution 3
Actually, the multipart data like image/zip or some other blob data will be included in $_FILES array, not $_POST array.
I recommend you to use the upload library.
view:upload_form.php
<html>
<head>
<title>Upload Form</title>
</head>
<body>
<?php echo $error;?>
<?php echo form_open_multipart('upload/do_upload');?>
<input type="file" name="userfile" size="20" />
<br /><br />
<input type="submit" value="upload" />
</form>
</body>
</html>
view:upload_success.php
<html>
<head>
<title>Upload Form</title>
</head>
<body>
<h3>Your file was successfully uploaded!</h3>
<ul>
<?php foreach($upload_data as $item => $value):?>
<li><?php echo $item;?>: <?php echo $value;?></li>
<?php endforeach; ?>
</ul>
<p><?php echo anchor('upload', 'Upload Another File!'); ?></p>
</body>
</html>
controller:upload.php
<?php
class Upload extends CI_Controller {
function __construct()
{
parent::__construct();
$this->load->helper(array('form', 'url'));
}
function index()
{
$this->load->view('upload_form', array('error' => ' ' ));
}
function do_upload()
{
$config['upload_path'] = './uploads/';
$config['allowed_types'] = 'gif|jpg|png';
$config['max_size'] = '100';
$config['max_width'] = '1024';
$config['max_height'] = '768';
$this->load->library('upload', $config);
if ( ! $this->upload->do_upload())
{
$error = array('error' => $this->upload->display_errors());
$this->load->view('upload_form', $error);
}
else
{
$data = array('upload_data' => $this->upload->data());
$this->load->view('upload_success', $data);
}
}
}
?>
And that's all
Solution 4
Check for possible wrong redirection in CI layer. It could be caused by a wrong URL request (for example, a slash '/' missing at the end of the URL). Redirections delete any POST data (I was dealing with this).
So, be sure that you are using full URLs in form action.
Check for your .htaccess and $config['uri_protocol']
consistency too.
Solution 5
I just had the same problem. I solved it this way. in application/config/config.php you set the base_url please be aware of, that this url have to be exact the same as the one you're calling your web app. For me, I called my domain with www.sdfsd.com, but in the config file I wrote http://sdfsd.com and so the POST of the form didn't work. So I changed this and now everything is working!!
cheers
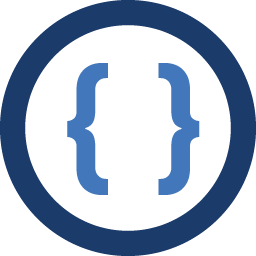
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
Im trying to make a file upload in CodeIgniter, how ever when I add enctype="multipart form-data" no post data will go through. At all not even the other fields. However when i dont add it, i can get the other post data, but of course no file upload. Whats going wrong here. Here is my view and controller:
View:
<h2>Add a New Album</h2> <form enctype="multipart/form-data" method="post" action="<?php echo base_url();?>index.php/photo/newAlbum"> <table style="margin-left:5px;"> <tr> <td> Album Name:</td> <td><input type="text" name="name" /></td> </tr> <tr> <td> Photo .zip File:</td> <td><input type="file" name="userfile" size="20" /></td> </tr> <tr> <td></td> <td><input type="submit" value="Upload Photo File" /></td> </tr> </table> </form>
controller only contains:
var_dump($_POST);
Result is:
array(0) { }
-
Basic over 12 yearsCodeIgniter unsets
$_POST
,$_GET
, etc... as a security measure and offers a managed way to access them via$this->input->get("Foo");
It seems the managed access isn't populated for multipart forms -
Marshall Davis over 12 yearsNote that your base URL must match the
action
attribute of forms if you are using the full URL. For example ifbase_url = http://www.mysite.com
and you use<form method="post" action="http://mysite.com/my_script">
you will receive an empty $_POST array. -
Marshall Davis over 12 yearsBy default it does not destroy the global $_GET array and it doesn't seem to ever unset the $_POST global. It can be made to destroy the $_GET array with
$config['allow_get_array'] = FALSE
but the $_POST array appears to remain intact. The input helper functions only simplify retrieval from these arrays by returning false if the index does not exist. Otherwise so long as $_POST['foo'] is set$this->input->post('foo')
and$_POST['foo']
are equivalent. CodeIgnitor User Guide Entry -
Abd Ul Aziz almost 10 yearsThanks @Mustafa, This solved a problem i've had for days now.