CodeIgniter load database in Controller or Model?
Solution 1
Go to autoload.php
in application/config/autoload.php
and add this
$autoload['libraries'] = array('database'); // add database in array(now you dont need to load database at anywhere in project)
Make database connection settings in database.php
, file located atapplication/config/database.php
now try this
class Users_model extends CI_Model {
function __construct() {
parent::__construct();
//$this->load->database(); <----remove this
}
public function get_all_users() {
return $this->db->get('users');
}
}
Solution 2
You can either load the database in the controller or you can load it in the model.
There's not much of a difference its just more neat and clean that all the interaction in the database is in the model and the controllers the one who connects both views and model.
The controller here is like a middleman between the buyer and the seller.
load database in the controller
<?php
class Test_controller extends CI_Controller {
public function __construct()
{
parent::__construct();
$this->load->database('default');
$this->load->model('test_model');
}
public function index()
{
$this->db->from('test');
$query = $this->db->get();
echo "<title>CodeIgniter SQlite</title>";
$data['db']=$query->result();
//print_r($data['db']);
$count = count($data['db']);
echo "Row count: $count rows<br>";
for ($i=0; $i < $count; $i++) {
echo $data['db'][$i]->text." ";
}
}
}
?>
load database in the model
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Test_model extends CI_Model
{
public $table1 = 'test';
public $table2 = '';
public function __construct()
{
parent::__construct();
$this->load->database('default');
}
public function check_db()
{
$this->db->from($this->table1);
$query = $this->db->get();
return $query->result();
}
}
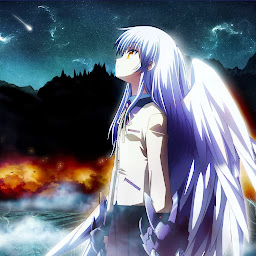
dango sjv
Updated on November 10, 2020Comments
-
dango sjv over 3 years
Model :
class Users_model extends CI_Model { function __construct() { parent::__construct(); $this->load->database(); } public function get_all_users() { return $this->db->get('users'); } }
Controller :
class Users extends CI_Controller { function __construct() { parent::__construct(); $this->load->helper('form'); $this->load->helper('url'); $this->load->helper('security'); $this->load->model('Users_model'); } public function index() { redirect('users/view_users'); } public function view_users() { $data['query'] = $this->Users_model->get_all_users(); $this->load->view('users/view_all_users', $data); } }
My question is where should i put the $this->load->database? In Model or Constructor? If possible tell me why? And one more question, if i omit the $this->load->database, the error shown
"Undefined property: Users::$db". I'm expecting "Undefined property: Users_model::$db".
Why is that? Is it looking for $db in both controller or model? Thank you. Note: i can connect to database just fine. What im actually ask is if i want to use $this->load->database(). Where should i put it? Controller or Model? And why?