Compare JSON response using JUnit and JSONassert
13,686
Solution 1
You can get the data as String
and pass it into JSONAssert.assertEquals
.
Converting to JSONObject
isn't necessary.
To fetch data from an URL, you can use URL.getContent
method:
final String data = new URL("...").getContent();
String expected = "{friends:[{id:123,name:\"Corby Page\"}"
+ ",{id:456,name:\"Solomon Duskis\"}]}";
JSONAssert.assertEquals(expected, data, false);
Solution 2
This can also be achieved with ModelAssert - https://github.com/webcompere/model-assert , which can take anything serializable to JSON as an input:
@Test
public void testGetFriends() throws JSONException {
JSONObject data = getRESTData("/friends/367.json");
String expected = "{friends:[{id:123,name:\"Corby Page\"}"
+ ",{id:456,name:\"Solomon Duskis\"}]}";
assertJson(data).isEqualTo(expected);
}
IIRC JSONObject
is essentially a Map
, so assertJson
will convert it to the internal JsonNode
format it uses for comparison.
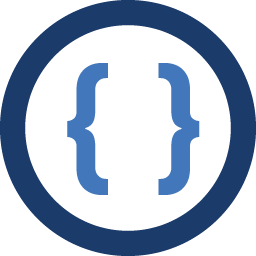
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm relatively new to Java and I'm asking to write test of JSON response server. I found JSONassert very useful but I didn't succeed to write the method
getRESTData
.Anybody can help please?
@Test public void testGetFriends() throws JSONException { JSONObject data = getRESTData("/friends/367.json"); String expected = "{friends:[{id:123,name:\"Corby Page\"}" + ",{id:456,name:\"Solomon Duskis\"}]}"; JSONAssert.assertEquals(expected, data, false); }