Comparing characters in strings
Solution 1
I would probably just iterate over both of them at the same time with zip and a list comprehension and then take length of the list:
a='HORSE'
b='TIGER'
words=zip(a,b)
incorrect=len([c for c,d in words if c!=d])
print(incorrect)
Zipping pairs lists together index-for-index, stopping when one runs out. List comprehensions are generators that are basically compact for-statements that you can add logic to. So it basically reads: for each zipped pair of letters (c,d) if c!=d then put a into the list (so if the letters are different, we increase the list length by 1). Then we just take the length of the list which is all the letters that are positionally different.
If we consider missing letters to be different, then we can use itertools.zip_longest to fill out the rest of the word:
import itertools
a='HORSES'
b='TIG'
words=itertools.zip_longest(a,b,fillvalue=None)
incorrect=len([c for c,d in words if c!=d]) ## No changes here
print(incorrect)
Obviously, None will never equal a character, so the difference in length will be registered.
EDIT: This hasn't been mentioned, but if we want case-insensitivity, then you just run .lower() or .casefold() on the strings beforehand.
Solution 2
sum([int(i!=j) for i,j in zip(a,b)])
would do the trick
Solution 3
use zip
to iterate over both strings consecutively
>>> def get_difference(str_a, str_b):
... """
... Traverse two strings of the same length and determine the number of
... indexes in which the characters differ
... """
...
... # confirm inputs are strings
... if not all(isinstance(x, str) for x in (str_a, str_b)):
... raise Exception("`difference` requires str inputs")
... # confirm string lengths match
... if len(str_a) != len(str_b):
... raise Exception("`difference` requires both input strings to be of equal length")
...
... # count the differences; this is the important bit
... ret = 0
... for i, j in zip(str_a, str_b):
... if i != j:
... ret += 1
... return ret
...
>>> difference('HORSE', 'TIGER')
5
also, the general style is to lower case function names (which are often verbs) and title case class names (which are often nouns) :)
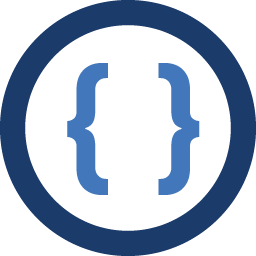
Admin
Updated on February 19, 2020Comments
-
Admin about 4 years
I'm trying to create a function that compares characters in the same position of two strings of same length and returns the count of their differences.
For instance,
a = "HORSE" b = "TIGER"
And it would return 5 (as all characters in the same position are different)
Here's what I've been working on.
def Differences(one, two): difference = [] for i in list(one): if list(one)[i] != list(two)[i]: difference = difference+1 return difference
That gives an error "List indices must be integers not strings"
And so I've tried turning it to int by using int(ord(
def Differences(one, two): difference = 0 for i in list(one): if int(ord(list(one)[i])) != int(ord(list(two)[i])): difference = difference+1 return difference
Which also returns the same error.
When I print list(one)[1] != list(two)[1] it eithers returns True or False, as the comparison is correctly made.
Can you tell me how to correct my code for this purpose?