Compile error in java :cannot find symbol.
Solution 1
Scanner input = new Scanner (inFile);
input
is local to the constructor, you cannot access outside of it, and you are trying to access in makeSmallerLists()
method. Make it as a instance member, So that it available through out the class
other than static
context.
public class Grocery{
Scanner input;
and in constructor
public Grocery(){
File inFile = new File ("lists.txt");
input = new Scanner (inFile);
Solution 2
You have variable scope problem. You can't get access a field outside of scope. Declare Scanner as globally, outside of costructor.
public class Grocery{
Scanner input = null;// Declare Scanner here.
public Grocery(){
.....
input=new Scanner (inFile);
}
Also append method bracket ()
.
public void makeSmallerLists(){
......
while(input.hasNextLine()){
line = input.nextLine();// Append () after method.
.....
}
Solution 3
That is because the Scanner object input
has been declared inside your constructor(local scope of the constructor) and thus its not visible in your makeSmallerLists()
. You need to declare it as an instance variable so that it would be accessible in all the methods of the class.
public class Grocery {
Scanner input; // declared here, as an instance variable
public Grocery(){
File inFile = new File ("lists.txt");
input = new Scanner (inFile); // initialized here
...
}
...
public void makeSmallerLists() {
...
while(input.hasNextLine()) { // accessible everywhere within the class
...
}
}
Solution 4
One solution is to have a class member of type Scanner
:
private Scanner input;
And in the constructor, construct it:
public class Grocery {
private Scanner input;
public Grocery() {
...
...
input = new Scanner (inFile);
}
...
}
Now input
is not limited to the scope of the constructor, it'll be accessible through the whole class.
Consider this example:
public class Example {
int n = 0; //n known everywhere in the class
if(n == 0) {
int n1 = 1;
if(n1 == 1) {
int n2 = 2;
//n1 is known here
}
//n2 is not known here
}
//n1 is not known here
}
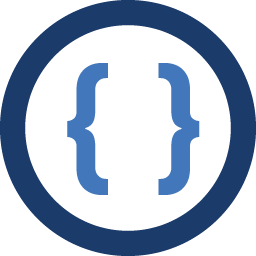
Admin
Updated on November 28, 2020Comments
-
Admin over 3 years
I have this code that is taking a text file and turning it into a string and then separating parts of the string into different elements of an arraylist.
import java.util.Scanner; import java.io.*; import java.util.ArrayList; public class Grocery{ public Grocery(){ File inFile = new File ("lists.txt"); Scanner input = new Scanner (inFile); String grocery; { grocery = input.nextLine(); } } public void makeSmallerLists(){ String listLine; String line; ArrayList<String> smallList = new ArrayList<String>(); while(input.hasNextLine()){ line = input.nextLine; if(line.equals("<END>")){ smallList.add(listLine); } else{ listLine = listLine + "\n" + line; } } } }
However when I try to compile this it gives me two errors:
javac Message.java Message.java:31: cannot find symbol symbol : variable input location: class Message while(input.hasNextLine()){ ^ Message.java:32: cannot find symbol symbol : variable input location: class Message line = input.nextLine; ^
How do I fix this? I really don't know what's wrong.
I fixed that and now my error says $ javac Message.java Message.java:34: cannot find symbol symbol : variable nextLine location: class java.util.Scanner line = input.nextLine; ^
^
Now what is wrong?