Compile in c++14
Solution 1
There's lots of change between C++ standards, so what is valid in one revision need not be in another.
g++ defaults to -std=gnu++98
for C++, which is the decades old C++98-standard enhanced with GNU extensions (most of which are conformant).
Choose the proper revision: -std=c++1y -pedantic
is a very close approximation to C++14.
What changes introduced in C++14 can potentially break a program written in C++11?
Solution 2
Looking at what you say you're having to use and the name format of that .cpp file, I think I'm in the same class. A year later, looks like, but here's my solution for archive's sake:
The std_lib_facilities.h header comes with the Bjarne Stroustrup textbook, "Programming: Principles and Practices Using C++". For those unaware, Bjarne Stroustrup invented C++ (he has a pretty good idea what he's talking about). Incidentally, the book is a fantastic way to learn C++, if one takes the time to actually read it. The std_lib_facilities.h header is just a convenient header file for beginners in C++, containing links to all the major standard libraries used in the textbook, as well as some helper functions that help account for potential mistakes or errors, or are just convenient for learning (such as an error() function that handles simple exception throwing for the student, or adding an "out of bounds" check for vectors). It's ultimately just a way to allow students to hop right into code without having to learn specifics about the header. Stroustrup keeps updated with C++ and thus includes several libraries that require the c++11 standard. The CSCE department wants its students (at least in this early class) to connect to the department's Unix system and compile from there, in order to avoid confusion with downloading and updating compilers.
I happened to already have had a couple C++ classes beforehand, and thus already had g++ set up on my Ubuntu laptop. I avoided including the std_lib_facilities for as long as possible since I was getting the same error as Topic Creator Joe, where g++ didn't recognize the "c++11" part (manually including the required libraries worked fine until we had to use a class from the textbook that used one of the header's helper functions) . Eventually, I found a help topic online that advised me simply to update my g++ compiler to 4.7 or higher, since 4.6 and lower doesn't have support for C++11 (or, of course, C++14). It was oddly rather involved compared to updates one might be used to on Mac or Windows, and I doubt the exact process would apply, but that is (was?) likely the problem: it's just an older version of g++, and it needs an update to compile C++11 and later. I recommend searching for ways to update g++/gcc for Mac.
Should, y'know, anyone else with this problem stumble upon this and not have their problem solved yet.
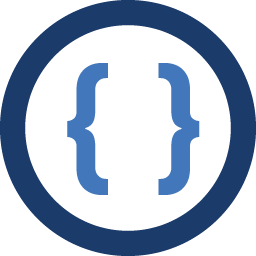
Admin
Updated on August 11, 2022Comments
-
Admin over 1 year
So in my CSE course we are given a header file to use right now for our programs that we're writing.
Unfortunately I can't get terminal to compile using that header, it gives quite a few errors (compiling with just 'g++'). Also, when I'm at my university and I'm using PuTTY I get the same errors while using this header. However, I don't get the errors when I compile with 'g++ -std=c++14'.
I've tried compiling with this command on terminal on my mac, but it says it doesn't recognize the c++14 part.
dhcp-10-202-147-243:hw1pr1 Admin$ g++ -std=c++14 hw1pr1.cpp error: invalid value 'c++14' in '-std=c++14'
Any help on how I could get this to work would be greatly appreciated. Hopefully this all made some sort of sense.
Here's the error I get when I compile with the header file I'm talking about in terminal with just g++.
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../lib/c++/v1/ext/hash_map:212:5: warning: Use of the header <ext/hash_map> is deprecated. Migrate to <unordered_map> [-W#warnings] # warning Use of the header <ext/hash_map> is deprecated. Migrate to ... ^ In file included from read_first_name.cpp:1: ./std_lib_facilities_4.h:43:20: error: no matching function for call to object of type 'hash<char *>' return hash<char*>()(s.c_str()); ^~~~~~~~~~~~~ /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../lib/c++/v1/ext/__hash:39:12: note: candidate function not viable: 1st argument ('const value_type *' (aka 'const char *')) would lose const qualifier size_t operator()(char *__c) const _NOEXCEPT ^ In file included from read_first_name.cpp:1: ./std_lib_facilities_4.h:112:8: warning: comparison of unsigned expression < 0 is always false [-Wtautological-compare] if (i<0||size()<=i) throw Range_error(i); ~^~ ./std_lib_facilities_4.h:118:8: warning: comparison of unsigned expression < 0 is always false [-Wtautological-compare] if (i<0||size()<=i) throw Range_error(i); ~^~ 3 warnings and 1 error generated.
This error doesn't happen and the program will compile fully when I use PuTTY and 'g++ std=c++14'