Compound class names not permitted error Webdriver
Solution 1
You can access the element if it has multiple classes using "By":
from selenium.webdriver.common.by import By
driver.findElement(By.cssSelector(".alert.alert-success"));
Solution 2
You can use
driver.findElement(By.className("alert-success"));
or
driver.findElement(By.className("alert"));
As right now selenium doesn't support multiple class name.If your class name includes a space, WebDriver will see it as a "compound selector". You can use cssSelector or id for selecting the webelement.
Solution 3
If usage of class name is must you can use the following ways:
1) css selectors:
driver.findElement(By.cssSelector(".alert.alert-success");
2) using xpath
driver.findElement(By.xpath("//div[@class='alert alert-success']"))
Try avoiding xpath and use css selectors instead.
Solution 4
It can also be done using class name like:
driver.find_element_by_class_name("alert")
or
driver.find_element_by_class_name("alert-success")
you can select anyone class name from two or more class names separated by spaces it'll work just fine.
Solution 5
The issue is because of the way find by Class name works.
in your code class name is class="alert alert-success"
If the class name has space you'll get the above error. You can simply get rid of the issue by using Id, CSS, Xpath, regular expression or any other element finder method.
Do you need to use Class Name or can you use another method? Let me know if you need to use class name.
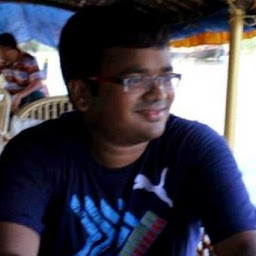
Ragunath Chilkuru
Updated on September 11, 2020Comments
-
Ragunath Chilkuru almost 4 years
I am getting the following error:
"Compound class names not permitted"
While trying to access web element where-in the element's class name have spaces in between. The page source for the web element is as below.
driver.findElement(By.className("alert alert-success"));
<div class="alert alert-success" alert-dismissable"="" id="58417" style="display: none;"> <button type="button" class="close hide-panel close-icon-58417" data-dismiss="alert" aria-hidden="true" style="display: inline-block;">×</button><span id="caret-58417" class="notification-caret caret-58417"></span> <div class="hide-panel close-icon-58417" id="58417" style="display: block;"> <span class="glyphicon glyphicon-ok-sign"></span><strong>Success</strong> KeyLinks Updated Successfully <div class="notification-panel-body panel-body-58417">REST Invocation Success</div> </div> </div>
I tried to find the element by CSS path as below. But the element is not searchable with this.
driver.findElement(By.cssSelector(".alert alert-success"));
This was the workaround given in the Link but still no success. your help will be much appreciated.