Compress all file .js with Google Closure Compiler Application in one File
Solution 1
java -jar path/to/closure-compiler/build/compiler.jar \
--js input_one.js \
--js input_two.js \
... \
--js_output_file compiled_output.js
Solution 2
I tried Orbits' answer, but It didn't really work perhaps the version I use is a newer version. The command I use is :
java -jar compiler.jar --js file1.js file2.js file3.js --js_output_file --js_output_file compiled_output.js.
Solution 3
Assuming that you’ve installed the Closure Compiler with Homebrew on a Mac, you can also concatenate all files and then pipe them to to Closure (this should also work the java -jar
way, but I haven’t verified it):
cat $(ls scripts/*.js) | closure-compiler --js_output_file main.js
Solution 4
Here are five globbing techniques for including multiple input files, with documentation extracted from the CommandLineRunner
class:
(1) This is a variation of muka's technique, removing the --js
flag, which is not needed:
java -jar compiler.jar \
--js_output_file build/out.js `find ./src/*.js`
From the docs:
The
--js
flag name is optional, because args are interpreted as files by default.
This will include all .js
files in /src/
, but won't include any files in subdirectories of /src/
.
(2) Similar to 1, but will include all .js
files in /src/
and all its subdirectories:
java -jar compiler.jar \
--js_output_file build/out.js `find ./src/ -name '*.js'`
(3) Similar to 2, but uses xargs
:
find ./src/ -name '*.js' \
| xargs java -jar compiler.jar \
--js_output_file build/out.js \
--manage_closure_dependencies
From the docs:
It is convenient to leverage the additional arguments feature when using the Closure Compiler in combination with
find
andxargs
:find MY_JS_SRC_DIR -name '*.js' \ | xargs java -jar compiler.jar --manage_closure_dependencies
The
find
command will produce a list of '*.js' source files in theMY_JS_SRC_DIR
directory whilexargs
will convert them to a single, space-delimited set of arguments that are appended to thejava
command to run the Compiler.Note that it is important to use the
--manage_closure_dependencies
option in this case because the order produced byfind
is unlikely to be sorted correctly with respect togoog.provide()
andgoog.requires()
.
(4) The v20140625
release added support for the **
(globstar) wildcard, which recursively
matches all subdirectories.
For example, this will include all .js
files in /src/
and all its subdirectories:
java -jar compiler.jar \
--js_output_file build/out.js './src/**.js'
More info here. From the docs:
You may also use minimatch-style glob patterns. For example, use:
--js='**.js' --js='!**_test.js'
to recursively include all js files that do not end in _test.js
From the Java docs:
The following rules are used to interpret glob patterns:
- The
*
character matches zero or more characters of a name component without crossing directory boundaries.- The
**
characters matches zero or more characters crossing directory boundaries.
(5) The v20140625
release also added a new feature: if the input path is a directory, then all .js
files
in that directory and all subdirectories will be included.
For example, this will include all .js
files in /src/
and all its subdirectories:
java -jar compiler.jar \
--js_output_file build/out.js './src/'
More info here.
Solution 5
You can use KjsCompiler: https://github.com/knyga/kjscompiler . Compiles multiple JavaScript files with Google Closure Compiler application in a right order
How to solve your problem: 1. Add annotations to js files, like this:
/**
* @depends {lib/somefile.js}
**/
If you do not care about compilation chain, you can ignore this step.
2. java -jar kjscompile.jar
You can look for example here: https://github.com/knyga/kjscompiler/tree/master/examples
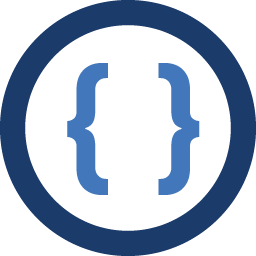
Admin
Updated on June 05, 2022Comments
-
Admin about 2 years
I would like to Compress all my file
.js
in a same directory in one file with Google Closure Compiler in a command line.For one file it's :
java -jar compiler.jar --js test.js --js_output_file final.js
But I didn't find in the doc how put my other file at the end of
final.js
without write over the last compress file ?I would like something like that :
java -jar compiler.jar --js --option *.js --js_output_file final.js
It's possible or must I do a programm who add all file in a file and after compress it ? Thank you if you can help me !