concatenate in swift: Binary operator '+' cannot be applied to operands of type 'String' and 'AnyObject'
The error message might be misleading in the first example
if currentUser["employer"] as! Bool == false { print("employer is false: "+currentUser["employer"] as! Bool) }
In this case, the error message is supposed to be
binary operator '+' cannot be applied to operands of type 'String' and 'Bool'
because currentUser["employer"] as! Bool
is a non-optional Bool
and cannot be implicitly cast to String
Those examples
print("employer: "+currentUser["employer"]) print("employer: \(currentUser["employer"])")
don't work because
- In the first line,
currentUser["employer"]
without any typecast is an optionalAnyObject
(aka unspecified) which doesn't know a+
operator. - In the second line, the string literal
"employer"
within the String interpolated expression causes a syntax error (which is fixed in Xcode 7.1 beta 2).
Edit:
This syntax is the usual way.
let isEmployer = currentUser["employer"]
print("isEmployer: \(isEmployer)")
Or alternatively, you can write
print("employer is " + String(currentUser["employer"] as! Bool))
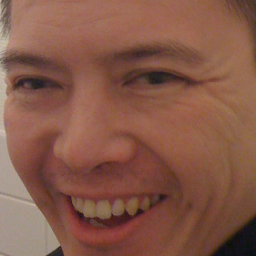
Tilo Delau
Best at java, pretty good at android, learning xcode and objective c.
Updated on June 16, 2022Comments
-
Tilo Delau almost 2 years
I get the error in Swift and don't understand it when I do this:
if(currentUser["employer"] as! Bool == false) { print("employer is false: "+currentUser["employer"] as! Bool) }
But I can do (Its not actually printing anything though, maybe another problem):
if(currentUser["employer"] as! Bool == false) { print(currentUser["employer"]) }
Results in error:
Binary operator '+' cannot be applied to operands of type 'String' and 'AnyObject'
Similarly:
let currentUser = PFUser.currentUser()! let isEmployer = currentUser["employer"] print("isEmployer: \(isEmployer)") print(currentUser["employer"])
But these two don't work:
print("employer: "+currentUser["employer"]) print("employer: \(currentUser["employer"])")
I also happen to be using Parse to get data, and not sure if that is the right way either.
-
Arthur Gevorkyan over 8 yearscould you specify the error?
-
Tilo Delau over 8 yearsThe error was in the title, exactly as is: Binary operator '+' cannot be applied to operands of type 'String' and 'AnyObject'
-
-
Tilo Delau over 8 yearsok. So last example will be a feature added in the future, but the first two are casting errors and is just something that can't be done with swift?
-
vadian over 8 yearsIt can be. Your code
let isEmployer = currentUser["employer"] print("isEmployer: \(isEmployer)")
is the proper way. Or you can writeprint("employer is " + String(currentUser["employer"] as! Bool))
-
Tilo Delau over 8 yearsprint("employer is " + String(currentUser["employer"] as! Bool)) is what I was looking for...which is too tedious! But now I clearly understand what is going on. So I should cast it myself in a variable first. With simple stuff, I've gotten away with using +. Thank you.