Concatenating arrays in Julia
Solution 1
Use the vcat
and hcat
functions:
julia> a, b = [1;2;3], [4;5;6]
([1,2,3],[4,5,6])
help?> vcat
Base.vcat(A...)
Concatenate along dimension 1
julia> vcat(a, b)
6-element Array{Int64,1}:
1
2
3
4
5
6
help?> hcat
Base.hcat(A...)
Concatenate along dimension 2
julia> hcat(a, b)
3x2 Array{Int64,2}:
1 4
2 5
3 6
Solution 2
Square brackets can be used for concatenation:
julia> a, b = [1;2;3], [4;5;6]
([1,2,3],[4,5,6])
julia> [a; b]
6-element Array{Int64,1}:
1
2
3
4
5
6
julia> [a b]
3×2 Array{Int64,2}:
1 4
2 5
3 6
Solution 3
You can use the cat
function to concatenate any number of arrays along any dimension. The first input is the dimension over which to perform the concatenation; the remaining inputs are all of the arrays you wish to concatenate together
a = [1;2;3]
b = [4;5;6]
## Concatenate 2 arrays along the first dimension
cat(1,a,b)
6-element Array{Int64,1}:
1
2
3
4
5
6
## Concatenate 2 arrays along the second dimension
cat(2,a,b)
3x2 Array{Int64,2}:
1 4
2 5
3 6
## Concatenate 2 arrays along the third dimension
cat(3,a,b)
3x1x2 Array{Int64,3}:
[:, :, 1] =
1
2
3
[:, :, 2] =
4
5
6
Solution 4
when encountered Array{Array,1}, the grammer is a little bit different, like this:
julia> a=[[1,2],[3,4]]
2-element Array{Array{Int64,1},1}:
[1, 2]
[3, 4]
julia> vcat(a)
2-element Array{Array{Int64,1},1}:
[1, 2]
[3, 4]
julia> hcat(a)
2×1 Array{Array{Int64,1},2}:
[1, 2]
[3, 4]
julia> vcat(a...)
4-element Array{Int64,1}:
1
2
3
4
julia> hcat(a...)
2×2 Array{Int64,2}:
1 3
2 4
ref:
... combines many arguments into one argument in function definitions In the context of function definitions, the ... operator is used to combine many different arguments into a single argument. This use of ... for combining many different arguments into a single argument is called slurping
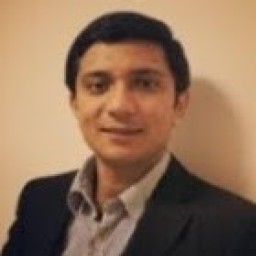
Abhijith
I am an Applied Mathematics professional working on machine learning algorithms(Collaborative Filtering) and using JULIA (julialang.org).
Updated on July 09, 2020Comments
-
Abhijith over 2 years
If the two
Int
arrays are,a = [1;2;3]
andb = [4;5;6]
, how do we concatenate the two arrays in both the dimensions? The expected outputs are,julia> out1 6-element Array{Int64,1}: 1 2 3 4 5 6 julia> out2 3x2 Array{Int64,2}: 1 4 2 5 3 6
-
HarmonicaMuse over 6 yearsThis is syntactic sugar for
vcat
andhcat
respectively:[e.head for e in [:([a; b]), :([a b])]] # Symbol[:vcat,:hcat]
-
Chris Rackauckas over 6 yearsGenerally I think
vcat
andhcat
should be preferred because this solution is whitespace sensitive. For example:[a - b]
willvcat
while[a -b]
willhcat
. That can be a nasty bug to find. -
DNF over 6 yearsIt seems a bit backward to not prefer the syntactic sugar version. After all, what's the sugar for? Are you saying that this syntax will probably be removed?
-
dividebyzero over 2 yearsMore recent versions would require the
dims
keyword, e.g.cat(a,b,dims=3)