Conditional SUM on oracle SQL
28,999
Solution 1
You can use SUM(CASE ... )
:
SELECT item, location,
SUM(CASE WHEN quantity > 0 THEN quantity ELSE 0 END) AS positive_sum,
SUM(CASE WHEN quantity < 0 THEN quantity ELSE 0 END) AS negative_sum
FROM your_table
GROUP BY item, location;
Solution 2
You can use GREATEST
and LEAST
in conjunction with the SUM
function:
Oracle 11g R2 Schema Setup:
CREATE TABLE table_name ( ITEM, LOCATION, QUANTITY ) AS
SELECT 100, 'KS', -10 FROM DUAL
UNION ALL SELECT 100, 'KS', -10 FROM DUAL
UNION ALL SELECT 100, 'KS', -20 FROM DUAL
UNION ALL SELECT 100, 'KS', 10 FROM DUAL
UNION ALL SELECT 100, 'KS', 5 FROM DUAL
UNION ALL SELECT 200, 'KS', 10 FROM DUAL
UNION ALL SELECT 200, 'KS', 20 FROM DUAL
UNION ALL SELECT 200, 'KS', 5 FROM DUAL
Query 1:
SELECT item,
location,
SUM( GREATEST( quantity, 0 ) ) AS positive_quantities,
SUM( LEAST( quantity, 0 ) ) AS negative_quantities
FROM table_name
GROUP BY item, location
| ITEM | LOCATION | POSITIVE_QUANTITIES | NEGATIVE_QUANTITIES |
|------|----------|---------------------|---------------------|
| 100 | KS | 15 | -40 |
| 200 | KS | 35 | 0 |
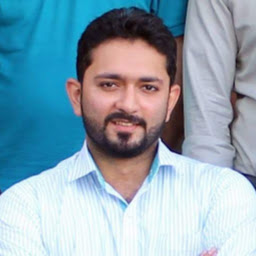
Author by
Imran Hemani
Updated on October 21, 2020Comments
-
Imran Hemani over 3 years
I have the data in the follow way:
ITEM LOCATION UNIT RETAIL QUANTITY 100 KS 10 -10 200 KS 20 30
I want the sum of positive quantities (quantity > 0) and sum of negative quantities (quantity < 0).
How do I get those column sum based on condition?
-
Lukasz Szozda over 8 yearsI like the idea of hiding
CASE
withGREATEST/LEAST
:) Could you compare execution plans with my solution? -
MT0 over 8 years@lad2025 Unsurprisingly - they both have identical plans. If you wish to profile them you can see if they have different performance (but I doubt it as they do exactly the same thing). Ultimately it comes down to which is more readable/maintainable/easier to type/etc...