Configure elasticsearch mapping with java api
21,364
Solution 1
I have successfully applied mappings to an Elasticsearch index using the Java API like the following:
XContentBuilder mapping = jsonBuilder()
.startObject()
.startObject("general")
.startObject("properties")
.startObject("message")
.field("type", "string")
.field("index", "not_analyzed")
.endObject()
.startObject("source")
.field("type","string")
.endObject()
.endObject()
.endObject()
.endObject();
PutMappingResponse putMappingResponse = client.admin().indices()
.preparePutMapping("test")
.setType("general")
.setSource(mapping)
.execute().actionGet();
Hope this helps.
Solution 2
Adding this for future readers. Please note you need to perform mapping prior to create the actual index or you will get an exception. See following code.
client.admin().indices().create(new CreateIndexRequest("indexname")).actionGet();
PutMappingResponse putMappingResponse = client.admin().indices()
.preparePutMapping("indexname")
.setType("indextype")
.setSource(jsonBuilder().prettyPrint()
.startObject()
.startObject("indextype")
.startObject("properties")
.startObject("country").field("type", "string").field("index", "not_analyzed").endObject()
.endObject()
.endObject()
.endObject())
.execute().actionGet();
IndexResponse response1 = client.prepareIndex("indexname", "indextype")
.setSource(buildIndex())
.execute()
.actionGet();
// Now "Sri Lanka" considered to be a single country :)
SearchResponse response = client.prepareSearch("indexname"
).addAggregation(AggregationBuilders.terms("countryfacet").field("country")).setSize(30).execute().actionGet();
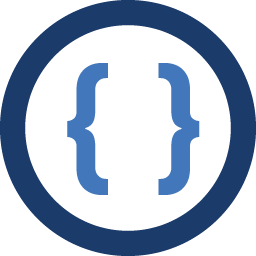
Author by
Admin
Updated on September 27, 2020Comments
-
Admin over 3 years
I have a few elasticsearch fields that I don't want to analyze before indexing. I have read that the right way to do this is by altering the index mapping. Right now my mapping looks like this:
{ "test" : { "general" : { "properties" : { "message" : { "type" : "string" }, "source" : { "type" : "string" } } } } }
And I would like it to look like this:
{ "test" : { "general" : { "properties" : { "message" : { "type" : "string", "index" : "not_analyzed" }, "source" : { "type" : "string" } } } } }
I have been trying to change the settings via
client.admin().indices().prepareCreate("test") .setSettings(getGrantSettings());
Where getGrantSettings() looks like:
static Settings getGrantSettings(){ JSONObject settingSource = new JSONObject(); try{ settingSource.put("mapping", new JSONObject() .put("message", new JSONObject() .put("type", "string") .put("index", "not_analyzed") )); } catch (JSONException e){ e.printStackTrace(); } Settings set = ImmutableSettings.settingsBuilder() .loadFromSource(settingSource.toString()).build(); return set; }