Configuring Maven to generate output outside the project directory
Solution 1
@khmarbaise is correct, with maven you are better off following conventions where possible - otherwise you should use a different build tool or you end up fighting it the whole way. That said, you can do the following to achieve your goal:
<build>
<outputDirectory>${user.home}/${project.artifactId}/target</outputDirectory>
...
</build>
This uses the user.home
system property as the root directory, and creates the output directory in a folder named after the <artifactId/>
('project'). All of the Java system properties are available in the pom. If you need this to be created elsewhere, you can just make it relative to the directory above.
Using maven-war-plugin instead:
Since your question indicates that you really just need the artifact (war file) output to a different directory, I suggest that you modify the configuration for the output directory in the maven-war-plugin. For example:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>2.0</version>
<configuration>
<outputDirectory>${user.home}/${project.artifactId}</outputDirectory>
</configuration>
</plugin>
</plugins>
</build>
Solution 2
The first thing you have to learn about Maven is: Maven has it's conventions one of those conventions is having a target folder which is located under the current project and not somewhere else. And furthermore and absolute no-go is putting some absolute folder definitions into your pom.
Solution 3
Very similar with the accepted answer, I am using a deployments folder which is mounted in a docker
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<configuration>
<outputDirectory>${project.build.directory}/deployments</outputDirectory>
</configuration>
</plugin>
</plugins>
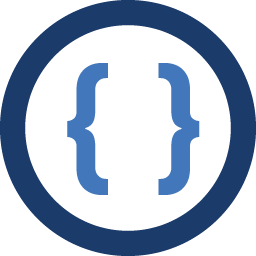
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
Please help me I am new to Maven. I am trying to generate a target folder in different directory from the Maven project folder. According to my requirements, the generated war file should be placed in another folder like C:\naresh folder when I build my maven project.
Here my code:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <packaging>war</packaging> <modelVersion>4.0.0</modelVersion> <groupId>com.test</groupId> <artifactId>project</artifactId> <version>1.0</version> <build> <directory>${project.basedir}/target</directory> <outputDirectory>C:\Software\${project.basedir}/target</outputDirectory> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <configuration> <warSourceDirectory>WebContent</warSourceDirectory> </configuration> </plugin> </plugins> </build> </project>