Confused with FromBody in ASP.NET Core
Solution 1
For anyone seeing this issue .net core 3 - you need to add the [ApiController] to the controller where you extend ControllerBase. The [FromBody] is only needed if you're doing an MVC controller.
This causes the body to get automatically processed in the way you're expecting.
Microsoft documentation for the ApiController attribute
Solution 2
And here's an alternate approach assuming you need to support both [FromForm]
and [FromBody]
in your Controller API…
Front-End (Angular Code):
forgotPassword(forgotPassword: ForgotPassword): Observable<number> {
const params = new URLSearchParams();
Object.keys(forgotPassword).forEach(key => params.append(key, forgotPassword[key]));
return this.httpClient.post(`${this.apiAuthUrl}/account/forgotpassword`, params.toString(), { headers: { 'Content-Type': 'application/x-www-form-urlencoded' } });
}
Back-End (C# Code):
[AllowAnonymous]
[HttpPost("[action]")]
public async Task<IActionResult> ForgotPassword(ForgotPasswordViewModel model) { }
Now your signature can remain the same so it can support both.
And another more permanent approach I thought about while addressing.
https://benfoster.io/blog/aspnet-core-customising-model-binding-conventions.
Hope it helps someone!
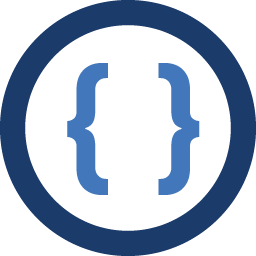
Admin
Updated on September 30, 2020Comments
-
Admin over 3 years
I have the following WEB API method, and have a SPA template with Angular:
[HttpPost] public IActionResult Post([FromBody]MyViewModel model)
I thought, based on this topic, there is no need to use
[FromBody]
here, since I want to read the value from the message body, so there is no need to override the default behavior, but, if I don't use[FromBody]
, the model that is coming from Angular is null. I'm really confused, why should I use[FromBody]
, since I have used the default behavior? -
Mathias Haugsbø over 4 yearsI have seen the same problem in two projects, is there something I missed in the migration documentation from 2.1 -> 2.2 -> 3.0 -> 3.1
-
xSx over 4 yearsare it mean i need add [ApiController] in any route O_o it's so much!(
-
duyn9uyen about 4 yearsI was looking all over for this! Thanks. I have a .NET Core Web API 3.1 project and didn't add [ApiController] to my controller. Then I needed [FromBody]. Added [ApiController] now it works without [FromBody].
-
Onur Omer over 3 yearsI owe you a complete-day! You've saved me!!
-
Daniel Frost about 3 yearsThis is not correct. It has to do with which content-type is being used. In order to bind the JSON correctly in ASP.NET Core, you must modify your action to include the attribute [FromBody] on the parameter. This tells the framework to use the content-type header of the request to decide which of the configured IInputFormatters to use for model binding. andrewlock.net/model-binding-json-posts-in-asp-net-core