Connect JS client with Python server
Solution 1
If you want to connect to the python TCP server, then you can use a code similar to the following in node:
var net = require('net');
var client = new net.Socket();
client.connect(8484, '127.0.0.1', function() {
console.log('Connected');
client.write('Hello, server! Love, Client.');
});
client.on('data', function(data) {
console.log('Received: ' + data);
client.destroy(); // kill client after server's response
});
The server started by your python code doesn't listens for raw TCP connections. If you read up on HTTP protocol you will understand why you saw those strings when your js code sent that string.
Solution 2
socket.socket()
doesn't create a websocket server.
You should use a websocket server, check this : https://websockets.readthedocs.io/en/stable/
Also your python client should use websockets
too, even though you could still send data using raw sockets.
Related videos on Youtube
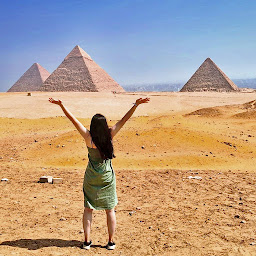
DidiNa
Updated on June 04, 2022Comments
-
DidiNa almost 2 years
I'm relatively new to JS and Python, so this is probably a beginners question. I'm trying to send a string from a JS client to Python Server (and then send the string to another Python client).
This is my code:
JS client:
var socket = io.connect('http://127.0.0.1:8484'); socket.send('lalala');
Python server:
HOST = '127.0.0.1' PORT = 8484 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.bind((HOST, PORT)) s.listen(2) #JS conn1, addr1 = s.accept() print 'Connected by', addr1 #PY conn2, addr2 = s.accept() print 'Connected by', addr2 while 1: try: data = conn1.recv(1024) except socket.error: print '' if data: print data.decode('utf-8') conn2.send('data')
Python client:
def __init__(self): #inicializacion self.comando = '0' self.HOST = '127.0.0.1' self.PORT = 8484 self.cliente = socket.socket(socket.AF_INET, socket.SOCK_STREAM) fcntl.fcntl(self.cliente, fcntl.F_SETFL, os.O_NONBLOCK) def activate(self): print "Plugin de envio en marcha." def deactivate(self): print "Plugin de envio off." def __call__(self, sample): self.cliente.connect((self.HOST, self.PORT)) try: self.comando = self.cliente.recv(1024).decode('utf-8') except socket.error, e: self.comando = '0' print "******************" print self.comando print "******************"
I have no problem sending a random string from python server to python client, but I can't receive from the JS client.
When I run the server and the clients there are no problems with the connection:
Connected by ('127.0.0.1', 52602) Connected by ('127.0.0.1', 52603)
But, for example, this is what I receive from the JS:
GET /socket.io/1/?t=1472322502274 HTTP/1.1 Host: 127.0.0.1:8484 Connection: keep-alive Origin: http://127.0.0.1:8880 User-Agent: Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.84 Safari/537.36 Accept: */* Referer: http://127.0.0.1:8880/normal.html Accept-Encoding: gzip, deflate, sdch Accept-Language: es-ES,es;q=0.8 Cookie: io=e7675566ceab4aa1991d55cd72c8075c
But I want the string 'lalala'.
Any ideas?
(And thank you!)
-
DidiNa over 7 yearsThank you! But I have this problem now: required.js:417 Uncaught Error: Module name "net" has not been loaded yet for context: _. Use require([]) requirejs.org/docs/errors.html#notloaded. I tried to solve it, but if I use: require(['net'], function (net) { }); I have: required.js:2417 GET 127.0.0.1:8880/net.js 500 (Internal Server Error) VM1275:1 Uncaught SyntaxError: Unexpected identifier and: required.js:417 Uncaught Error: Load timeout for modules: net requirejs.org/docs/errors.html#timeout Any ideas?
-
Nehal J Wani over 7 years@DidiNa Are you doing this in a browser?
-
DidiNa over 7 yearsYes, I have a web app where the user write a string, then: html -> js -> node.js -> python server -> python client. My problem now is send the string from js to node.js.
-
Nehal J Wani over 7 yearsOver which protocol is the
js
in browser gonna contact with the server runningnode.js
? -
DidiNa over 7 yearsI'm not sure, but Socket.IO uses the WebSocket protocol.