Connecting my iPad app to a SQL Server
I am currently working in an iOS application that requires this same functionality. For mySQL database queries on the server, I am using server-side PHP scripts that can accept variables, such as the table name or database search term.
What I do is I make an HTTP GET request using objective-C's NSMutableURLRequest, then have the server process the request (in PHP), and then return the database query results to my application in JSON format. I use SBJsonParser to parse the returned data into an NSData, and then an NSArray object.
An example of making an HTTP request in Objective-C:
NSString *urlString = [NSString stringWithFormat:@"http://website.com/yourPHPScript.php?yourVariable=something"];
NSURL *url = [NSURL URLWithString: urlString];
NSMutableURLRequest *request1 = [[NSMutableURLRequest alloc] initWithURL:url];
/* set the data and http method */
[request1 setHTTPMethod:@"GET"];
[request1 setHTTPBody:nil];
/* Make the connection to the server with the http request */
[[NSURLConnection alloc] initWithRequest:request1
delegate:self];
There is more code that you need to add to actually respond to the request when it returns, and I can post an example of that if you would like.
I actually dont know if this is the best way to do this, but It has worked for me so far. It does require that you know PHP though, and I don't you if you have any experience with it.
UPDATE:
Here is some sample code showing how to respond to the request. In my case, since I am getting a JSON encoded response, I use the SBJsonParser to parse the response.
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
/* This string contains the response data.
* At this point you can do whatever you want with it
*/
NSString *responseString = [[NSString alloc] initWithData:receivedData
encoding:NSUTF8StringEncoding];
/* Here I parse the response JSON string into a native NSDictionary using an SBJsonParser */
SBJsonParser *parser = [[[SBJsonParser alloc] init] autorelease];
/* Parse the JSON into an NSDictionary */
NSDictionary *responseArr = [parser objectWithString:responseString];
/* Do whatever you want to do with the response */
/* Relsease the connection unless you want to re-use it */
[connection release];
}
Also add these methods, assuming you have an NSMUtableData instance variable titled receivedData.
- (void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response
{
[receivedData setLength:0];
}
- (void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data
{
[receivedData appendData:data];
}
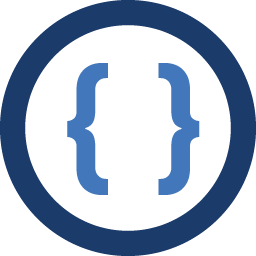
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I have an iPad application that uses table view controllers to present lists of data. I want to connect these tables to data in a SQL Server. I have very little experience in this realm of programming and I am not sure where to begin. In the long run I'd like adding/editing/deleting data on the app to sync with the server as well. I understand that this is a very broad question, so I am mainly looking for suggestions to help me get started. I, for example, do not want to start researching and learning Core Data if it is not the framework that can accomplish my goal.
In short, how can I connect my application to a SQL Server so that I can access its data and sync it to a device? Any example code/walkthroughs would be much appreciated.
-
bddicken almost 12 yearsOk cool, no worries. If you are going to be working with mySQL servers a lot in the future, I would definitely recommend learning it sometime. PHP is very valuable for situations like these!
-
Admin almost 12 yearsHi, I know this was from a long time ago...But I would like to see sample code of the NSURLResponse.
-
Admin almost 12 yearsYes it does thank you very much :) Although I am receiving XML and parsing it into a core data object, so I'm guessing I'm going to have to make my own parser instead of using a pre-made one?
-
bddicken almost 12 yearsI would try to find one that is already out there, it will save you a lot of time. Maybe this question will help.
-
Admin almost 12 yearsThanks again. That question has a lot of great links
-
bddicken almost 12 yearsThe server that I connect to is using the Rest api server-side, but I handle all iOS side networking with NSURLRequest's. I have never tried using RestKit, but it looks pretty cool.