Connecting two computers(machines over a wireless network)
To create client socket you need to use the server's IP address not yours.
package Connect1;
import java.io.*;
import java.net.*;
import javax.swing.JOptionPane;
import java.util.Scanner;
class TCPClient {
public static void main(String argv[]) throws Exception
{
String sentence;
String modifiedSentence;
BufferedReader inFromUser =new BufferedReader(new InputStreamReader(System.in));
Socket clientSocket = new Socket(InetAddress.getByName("SERVER_IP"), 6789);
DataOutputStream outToServer =new DataOutputStream(clientSocket.getOutputStream());
BufferedReader inFromServer =new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
System.out.print("Enter characters to be capitalized: ");
sentence =inFromUser.readLine();
outToServer.writeBytes(sentence + '\n');
modifiedSentence = inFromServer.readLine();
System.out.println("FROM SERVER: " + modifiedSentence);
Scanner input=new Scanner(System.in);
//System.out.print("Do you want to enter again? Press '0' for 'yes' and '1' if 'No'.");
//i=input.nextInt();
}
}
replace "SERVER_IP" by server's IP.
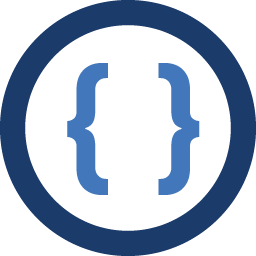
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I'm sitting in a room with my laptop which is connected to a wireless network. Beside me, my friend is sitting with another laptop which is also connected to the same network.
We want to connect our laptops using socket programming(eclipse/netbeans). How can we do this? This is what we have done so far:
- I have a client class on my laptop. And my friend has the server class on
hers (with my laptop's IP in the address). - We have disabled the firewalls on both laptops.
- We have open the port 6789 manually on both the laptops.
- We then firstly run the server class on my laptop and then run the client
class on hers.
These are the classes.
Server:
package Connect1; import java.io.BufferedReader; import java.io.DataOutputStream; import java.io.InputStreamReader; import java.net.ServerSocket; import java.net.Socket; import java.io.*; import java.net.*; public class Server1 { public static void main(String argv[]) throws Exception { String clientSentence; String capitalizedSentence; ServerSocket welcomeSocket = new ServerSocket(6789); while(true) { Socket connectionSocket = welcomeSocket.accept(); BufferedReader inFromClient = new BufferedReader(new InputStreamReader(connectionSocket.getInputStream())); DataOutputStream outToClient = new DataOutputStream(connectionSocket.getOutputStream()); clientSentence = inFromClient.readLine(); capitalizedSentence = clientSentence.toUpperCase() + '\n'; outToClient.writeBytes(capitalizedSentence); connectionSocket.close(); // welcomeSocket.close(); } } }
Client:
package Connect1; import java.io.*; import java.net.*; import javax.swing.JOptionPane; import java.util.Scanner; class TCPClient { public static void main(String argv[]) throws Exception { String sentence; String modifiedSentence; BufferedReader inFromUser =new BufferedReader(new InputStreamReader(System.in)); Socket clientSocket = new Socket("IP of my laptop here", 6789); DataOutputStream outToServer =new DataOutputStream(clientSocket.getOutputStream()); BufferedReader inFromServer =new BufferedReader(new InputStreamReader(clientSocket.getInputStream())); System.out.print("Enter characters to be capitalized: "); sentence =inFromUser.readLine(); outToServer.writeBytes(sentence + '\n'); modifiedSentence = inFromServer.readLine(); System.out.println("FROM SERVER: " + modifiedSentence); Scanner input=new Scanner(System.in); //System.out.print("Do you want to enter again? Press '0' for 'yes' and '1' if 'No'."); //i=input.nextInt(); } }
We get this error:
Exception in thread "main" java.net.ConnectException: Connection refused: connect at java.net.PlainSocketImpl.socketConnect(Native Method) at java.net.PlainSocketImpl.doConnect(Unknown Source) at java.net.PlainSocketImpl.connectToAddress(Unknown Source) at java.net.PlainSocketImpl.connect(Unknown Source) at java.net.SocksSocketImpl.connect(Unknown Source) at java.net.Socket.connect(Unknown Source) at java.net.Socket.connect(Unknown Source) at java.net.Socket.<init>(Unknown Source) at java.net.Socket.<init>(Unknown Source) at Code1.TCPClient.main(TCPClient.java:18)
Can anyone please tell me what we are doing wrong? Is there something missing in our code or procedure? Do we need to establish some other kind of connection first as well? Do we have to open other ports for doing this? Can this be done with different versions of eclipse on the two laptops?
- I have a client class on my laptop. And my friend has the server class on