Constructor in PHP
25,377
Solution 1
The PHP constructor can take parameters like the other functions can. It's not required to add parameters to the __construct()
function, for example:
Example 1: Without parameters
<?php
class example {
public $var;
function __construct() {
$this->var = "My example.";
}
}
$example = new example;
echo $example->var; // Prints: My example.
?>
Example 2: with parameters
<?php
class example {
public $var;
function __construct($param) {
$this->var = $param;
}
}
$example = new example("Custom parameter");
echo $example->var; // Prints: Custom parameter
?>
Solution 2
__construct
can take parameters. According to the official documentation, this method signature is:
void __construct ([ mixed $args = "" [, $... ]] )
So it seems it can take parameters!
How to use it:
class MyClass {
public function __construct($a) {
echo $a;
}
}
$a = new MyClass('Hello, World!'); // Will print "Hello, World!"
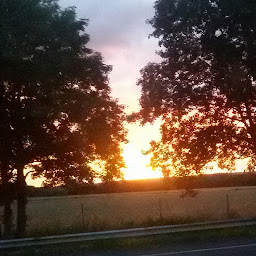
Author by
Blueberry
Updated on February 24, 2020Comments
-
Blueberry about 4 years
Does a constructor method in PHP take the parameters declared in the class or not?
I've seen on multiple sites and books and in the PHP documentation that the function function __construct() doesn't take any parameters.