Controller not working in mvc 4
Solution 1
Here are the following things you need to cross check
- Inheriting controller from Controller and not from ApiController
Make sure Routeconfig has proper Maproute
Else you need to rename the controller [sounds weird but it might help]
and use ActionResult as return datatype
Solution 2
A method in a Controller that returns a string
will not be routable. It must return an ActionResult
(or one of it's derived classes).
Change it to:
public ActionResult Home()
{
return View("Hello from home");
}
Then in your Views/Home folder, add a view called Home that has something like:
@model string
<p>@Model</p>
Solution 3
Things to consider:
- Make sure you're inheriting your controller from Controller and not from ApiController
- Make sure your routes are OK
- From the comments, also make sure if your controller is not Home, that User is default on your route
These are fairly simple things, but I've clicked OK several times just to realize that I selected ApiController on Asp .NET MVC 4!
Example route would be:
routes.MapRoute(
"Default", // Route name
"{controller}/{action}/{id}", // URL with parameters
new { controller = "User", action = "home", id = UrlParameter.Optional }
);
Please note that this will use UserController as default and home as the default view, although, I would recommend naming home to Index as it's more common.
If is not any of these, please post your whole controller so we can get a further look of it!
Hope I can help!
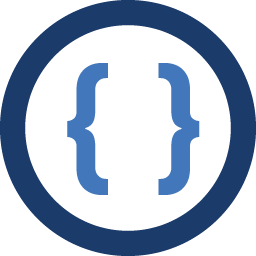
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I have a controller named as UserController and in that only Index action is being called another action added like as 'home action' is not being called and either calling actionresult/string or returning view
I am getting this error
while running at Google Chrome
{"Message":"No HTTP resource was found that matches the request URI /user/home'.","MessageDetail":"No type was found that matches the controller named 'home'."}
And running at Mozilla Firefox
Whoops! The page could not be found. Try giving it another chance below.
Here is my whole Controller Code:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace Mvc4Application1.Controllers { public class UserController : Controller { // // GET: /User/ public ActionResult Index() { return View(); } public ActionResult AddPlace() { return View(); } public ActionResult UpdatePlace() { return View(); } public ActionResult DeletePlace() { return View(); } } }
Here is RouteConfig.cs file
routes.MapRoute( name: "Default", url: "{controller}/{action}/{id}", defaults: new { controller = "Home", action = "Index", id=UrlParameter.Optional }