Conversion from char to int and printing the decimal value
Solution 1
There are two ways you could modify your code to do what you want. The first, would be to change the printf to print the character representation rather than the integer representation of the value.
printf("%c", str[i]);
Will print "0" given the in put of the string "0" from the keyboard.
The other one, which you mentioned in your question is:
printf("%d", (int)(str[i]-'0'));
The reason you get the correct answer here has to deal with how the computer stores values. When you type a "0", it doesn't actually store the value 0. It stores instead the ASCII representation of a character we call "0". That value happens to be the integer 48. 49 represents the character "1" and so forth.
Why? Well think about representing the character "M" or perhaps "#". What should represent those? ASCII is a known representation of 256 characters (roughly) since that's the total number of values we can store in 1 byte. So, "M" is 77 and "#" is 35.
The reason str[i] - '0' works has to do with the way C/C++ handles characters ('0' is a character). The character 'M' is exactly equivalent to the value 77. So these two lines would be equivalent:
str[i] - '0'
is the same as
str[i] - 65
Because I enter a "1" (string) which is represented in the binary as a 66, I subtract 65 and I get 1, the value. When I ask printf to display the integer value (%d) of my result
printf("%d", (int)(str[i] - '0'));
it prints "1" because 66 - 65 is 1. Or '1' - '0' is 1.
Just remember that any time you're looking at a string or a character you aren't looking at the digital or binary representation of a value, you're looking at the ASCII representation of that value.
Solution 2
If str[i]
is known to be a digit, (int)(str[i]-'0')
or more simply str[i] - '0'
is the value of this digit between 0
and 9
.
The reason for this: the C Standard mandates that the characters for representing '0'
, '1'
, ..., '9'
be exactly consecutive. Hence '1' - '0' == 1
, and so on for all digits.
Solution 3
Just because it has not been addressed yet...
Don't forget about the other way to convert char arrays to integer or float:
char num1[]="1234";
char num2[]="123.5";
int x = atoi(num1);
double y = atof(num2);
printf("%d\n", x);
printf("%f\n", y);
Note: strtof(,,) is another option for string to float converstion.
and strtol(,,) is another option for converting string to long int.
Solution 4
In your code,
printf("%d",(int)str[i]);
prints the ASCII value of the corresponding char
value(digit).
OTOH,
printf("%d",(int)(str[i]-'0'));
prints the difference of the ASCII value of the char
and ASCII of 0
.
If you look at the ASCII table, char
representation from 0
to 9
are having consecutive values, so the difference of the particular char
with ASCII value of 0
equals to the decimal representation of any particular digit.
FWIW, the cast to (int)
is not required in this case, you can directly write
printf("%d",str[i]-'0);
to get the decimal value of that char
digit.
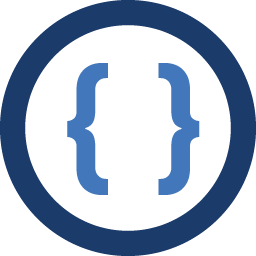
Admin
Updated on June 17, 2020Comments
-
Admin almost 4 years
char str[20]; printf("Enter anything\n"); scanf("%s",str); for(int i = 0 ; i<strlen(str) ; i++) { if( isdigit(str[i])) printf("%d",(int)str[i]); }
I tried with the above code but the output is in ASCII values of the numbers rather then actual numbers. Like , input: "0" output: "48".
When I try
(int)(str[i]-'0');
instead of(int)str[i]);
, I get the correct answer but I don't understand why it's correct.