Conversion of byte array containing hex values to decimal values
Solution 1
The BitConverter.ToInt32 method is a good way to do this
if (BitConverter.IsLittleEndian)
Array.Reverse(array); //need the bytes in the reverse order
int value = BitConverter.ToInt32(array, 0);
Solution 2
hex and decimal are just different ways to represent the same data you want something like
int myInt = array[0] | (array[1] << 8) | (array[2] << 16) | (array[3] <<24)
Solution 3
Here's a method to convert a string containing a hexadecimal number to a decimal integer:
private int HexToDec(string hexValue)
{
char[] values = hexValue.ToUpperInvariant().ToCharArray();
Array.Reverse(values);
int result = 0;
string reference = "0123456789ABCDEF";
for (int i = 0; i < values.Length; i++)
result += (int)(reference.IndexOf(values[i]) * Math.Pow(16d, (double)i));
return result;
}
You can then string the pieces together and pass them to this function to get the decimal values. If you're using very large values, you may want to change from int to ulong.
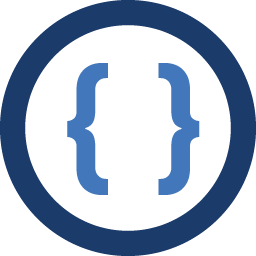
Admin
Updated on August 22, 2020Comments
-
Admin over 3 years
I am making application in c#.Here i want to convert a byte array containing hex values to decimal values.Suppose i have one byte array as
array[0]=0X4E; array[1]=0X5E; array[2]=0X75; array[3]=0X49;
Here i want to convert that hex array to decimal number like i want to concatenate first all bytes values as 4E5E7549 and after that conversion of that number to decimal.I dont want to convert each separate hex number to decimal.The decimal equivalent of that hex number is 1314813257.So please help me.Thanks in advance.