Convert List<List<T>> into List<T> in C#
15,891
Solution 1
List<List<int>> l = new List<List<int>>();
l.Add(new List<int> { 1, 2, 3, 4, 5, 6});
l.Add(new List<int> { 4, 5, 6, 7, 8, 9 });
l.Add(new List<int> { 8, 9, 10, 11, 12, 13 });
var result = (from e in l
from e2 in e
select e2).Distinct();
Update 09.2013
But these days I would actually write it as
var result2 = l.SelectMany(i => i).Distinct();
Solution 2
List<int> result = listOfLists
.SelectMany(list => list)
.Distinct()
.ToList();
Solution 3
How about:
HashSet<int> set = new HashSet<int>();
foreach (List<int> list in listOfLists)
{
set.UnionWith(list);
}
return set.ToList();
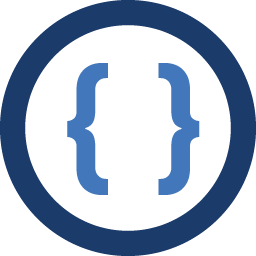
Author by
Admin
Updated on June 10, 2022Comments
-
Admin almost 2 years
I have a
List<List<int>>
. I would like to convert it into aList<int>
where each int is unique. I was wondering if anyone had an elegant solution to this using LINQ.I would like to be able to use the Union method but it creates a new List<> everytime. So I'd like to avoid doing something like this:
List<int> allInts = new List<int>(); foreach(List<int> list in listOfLists) allInts = new List<int>(allInts.Union(list));
Any suggestions?
Thanks!
-
Admin over 15 yearsThis is what I was looking for. Just add a .ToList() at the end of the Distinct and I have what I need. Thanks!