Convert list of dicts to string
Solution 1
', '.join(d['memberId'] for d in my_list)
Since you said you are new to Python, I'll explain how this works.
The str.join()
method combines each element of an iterable (like a list), and uses the string that the method is called on as the separator.
The iterable that is provided to the method is the generator expression (d['memberId'] for d in my_list)
. This essentially gives you each element that would be in the list created by the list comprehension [d['memberId'] for d in my_list]
without actually creating the list.
Solution 2
These one-liners are ok but a beginner might not get it. Here they are broken down:
list_of_dicts = (the list you posted)
Ok, we have a list, and each member of it is a dict. Here's a list comprehension
:
[expr for d in list_of_dicts]
This is like saying for d in list_of_dicts ...
. expr
is evaluated for each d and a new list is generated. You can also select just some of them with if
, see the docs.
So, what expr
do we want? In each dict d
, we want the value that goes with the key 'memberId'
. That's d['memberId']
. So now the list comprehension is:
[d['memberId'] for d in list_of_dicts]
This gives us a list of the email addresses, now to put them together with commas, we use join
(see the docs):
', '.join([d['memberId'] for d in list_of_dicts])
I see the other posters left out the [] inside join
's argument list, and it works. Have to look that up, I don't know why you can leave it out. HTH.
Solution 3
Access the dicts.
', '.join(d['memberId'] for d in L)
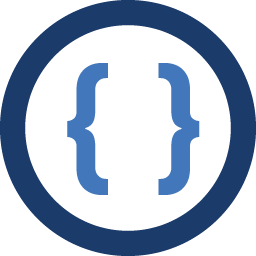
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
I'm very new to Python, so forgive me if this is easier than it seems to me.
I'm being presented with a list of dicts as follows:
[{'directMember': 'true', 'memberType': 'User', 'memberId': '[email protected]'}, {'directMember': 'true', 'memberType': 'User', 'memberId': '[email protected]'}, {'directMember': 'true', 'memberType': 'User', 'memberId': '[email protected]'}]
I would like to generate a simple string of memberIds, such as
but every method of converting a list to a string that I have tried fails because dicts are involved.
Any advice?