Convert size_t to string
Solution 1
You can use the members the sprintf()
-family of functions to convert "something" to a "string".
#define _POSIX_C_SOURCE 200112L
#include <stdio.h>
#include <unistd.h>
#include <string.h>
int main(void)
{
size_t s = 123456789;
char str[256] = ""; /* In fact not necessary as snprintf() adds the
0-terminator. */
snprintf(str, sizeof str, "%zu", s);
fputs(stdout, "The size is '");
fflush(stdout);
write(fileno(stdout), str, strlen(str));
fputs(stdout, "'.\n");
return 0;
}
Prints out:
The size is '123456789'.
Solution 2
You know that size_t
is unsigned, but the length is unknown. In C99, we have the z
modifier for that, making the complete specifier %zu
:
size_t s = 123456789;
char str[256];
snprintf(str, sizeof str, "%zu", s);
Solution 3
char *
is not necessarily a string, you can send what you want.
Just manage that the other computer use the same protocol.
so you can do that :
write(socket, &(buffer.st_size), sizeof(size_t));
this is maybe to fast, you may have to take account of the endianness etc.
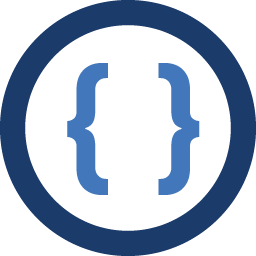
Admin
Updated on July 04, 2022Comments
-
Admin almost 2 years
I'm trying to write a TCP server which a client can use to browse the server's directories. In addition to that I want to send the size of the directory if that is a regular file. The size of the file is saved into a size_t variable under a "stat" struct. I'm doing this here:
char *fullName /* The path to the file *. /** * Some code here */ struct stat buffer; lstat(fullName, &buffer)
So now buffer.st_size contains the file's size. Now I want to write() it to the listening socket but apparently I have to convert it to a string somehow. I know this can be done somehow with the bitwise right-shift (>>) operator but it seems too painful to me. Can you help me out here (even if there's no way other that bitwise operators)?
By the way this is not for school or smth...
PS: I'm running this on Linux.
-
Fiddling Bits over 10 yearsFor someone who doesn't have the top of their head… you know your stuff! ;-)
-
Admin over 10 yearsI have bad experience with memory using sprintf() and snprintf(), however that's not exactly what I want and it's my fault I didn't explain this well. I actually want to convert the size of this into its scalings. e.g 123456789 would be written "117,73 MB". Now I know I can use the fact that the numbers 0-9 have the same ascii code for ints and strings but I don't know how to actually implement it. I have tried several things but I fail every time. Thanks for your input already.
-
Admin over 10 yearsIt has to be a string because it will be part of an HTML page.
-
alk over 10 years@user3119598: "I have bad experience with memory using sprintf() and snprintf() ..." it's all just a matter of training. Those functions are save and straight forward to use. They are far less dangerous than their use a for example their counterparts of the
scanf*()
-clan. Train to use thesprintf()
-functions as they are essential. -
Admin over 10 yearsI have used them but I usually get memory leaks in huge projects. Anyway it works in this one so thanks for the suggestion:). Now I want to convert the size_t from bytes to kb,mb etc.
-
hl037_ over 10 yearsOk, the "but apparently I have to convert it to a string somehow" has misled me ;)