Convert SOAP to REST
Solution 1
REST is a completely different way to think of a web service to SOAP. In particular, it operates in terms of resources, their representations and the links between them. (You also have HTTP verbs about, but for a simple query service like this one you'd probably only be using GET anyway.)
A RESTful version of that interface would work a bit like this:
- A client would decide they want to know some information about a particular place, so they'd ask the service to search for that place and tell them the link to it, or at least to places that match the search criteria; since that might be several different places (think "London, UK" vs. "London, Ont." in response to a search for "London") the result will be a page that links to the characterization of each of the places. It might also say a bit about what each link means, but that's not necessary. (The format of the result can be HTML, or XML, or JSON, or any of a number of different formats; HTTP content negotiation makes for a great way to pick between them.)
- Once the user has decided which place from the list they actually want information about, they follow the link and get a description of what information about that place is available. This is a document that provides links to a page that provides the max temperature, another page that provides the min temperature, and a third that provides the humidity.
- To get the actual data, another link is followed and that data is served up. Since the data is a simple float, getting it back as plain text is quite reasonable.
Now, we need to map these things to URLs:
- Searching:
/search?place=somename
(which is easy to hook up behind a place name), which contains links to… - Place:
/place/{id}
(where{id}
is some general ID that probably is your DB's primary key; we don't want to use the name here because of the duplicate name problem), which contains links to… - Data:
/place/{id}/maxTemp
,/place/{id}/minTemp
,/place/{id}/humidity
.
We also need to have some way to do the document creation. JAXB is recommended. Links should probably be done in XML with attributes called xlink:href
; using (by reference) the XLink specification like that states exactly that the content of the attribute is a link (the unambiguous statement of that is otherwise a real problem in XML due to its general nature).
Finally, you probably want to use JAX-RS to do the binding of your Java code to the service. That's way easier than writing it all yourself. That lets you get down to doing something like this (leaving out the data binding classes for brevity):
public class MyRestWeatherService {
@GET
@Path("/search")
@Produces("application/xml")
public SearchResults search(@QueryParam("place") String placeName) {
// ...
}
@GET
@Path("/place/{id}")
@Produces("application/xml")
public PlaceDescription place(@PathParam("id") String placeId) {
// ...
}
@GET
@Path("/place/{id}/maxTemp")
@Produces("text/plain")
public String getMaxTemperature(@PathParam("id") String placeId) {
// ...
}
// etc.
}
As you can see, it can go on a bit but it is not difficult to do so long as you start with a good plan of what everything means…
Solution 2
Thank goodness Donal Fellows pointed out the @WebService annotation. I didn't realize that this was a web service until he did.
You'll have more problems that API choices with this implementation. If you're deploying on a Java EE app server, I'd recommend using a JNDI data source and connection pool instead of hard wired database parameters and connecting for every request.
Better to close your resources in a finally block, wrapped in individual try/catch blocks.
Those empty catch blocks will drive you crazy. Bad things will happen, but you'll never know.
SOAP clients send an XML request over HTTP to a service that parses it, binds the values to inputs, uses them to fulfill the use case, and marshals a response as XML to send back.
REST asks clients to do all the same things, except instead of packaging the request parameters into an XML request they're sent via HTTP. The API is the HTTP GET, POST, DELETE. You express your method calls as URIs.
So it'll take a good knowledge of your SOAP API and some brains on your part. I don't know of any tools to do it for you.
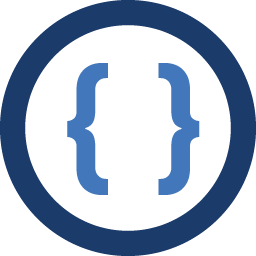
Admin
Updated on August 12, 2022Comments
-
Admin over 1 year
How to to Convert SOAP to REST using java language?
package net.weather; import java.sql.*; import javax.jws.WebService; @WebService public class ProjectFinalWS{ Connection con; Statement st; ResultSet rs; String res; public void connectDB() { String url ="jdbc:mysql://localhost:3306/"; String dbName ="project"; String driver = "com.mysql.jdbc.Driver"; String userName = "root"; String password = "root"; try { Class.forName(driver).newInstance(); con = DriverManager.getConnection(url+dbName,userName,password); }catch(Exception e){} } public float getMaxTemp(String city) { float mxtemp=0; connectDB(); try{ st=con.createStatement(); rs=st.executeQuery("select maxtemp from weather where city='"+city+"'"); rs.next(); mxtemp=rs.getFloat(1); st.close(); con.close(); } catch(Exception e){} return mxtemp; } public float getMinTemp(String city) { float mntemp=0; connectDB(); try{ st=con.createStatement(); rs=st.executeQuery("select mintemp from weather where city='"+city+"'"); rs.next(); mntemp=rs.getFloat(1); st.close(); con.close(); } catch(Exception e){} return mntemp; } public float getHumidity(String city) { float humidity=0; connectDB(); try{ st=con.createStatement(); rs=st.executeQuery("select humidity from weather where city='"+city+"'"); rs.next(); humidity=rs.getFloat(1); st.close(); con.close(); } catch(Exception e){} return humidity; } }
-
Morten Jensen over 11 yearsI agree. I don't think there is a tool to convert the implicit decorated stuff that seems to be going on with this java code. Best bet would be to abstract the SOAP-specifics away in a separate layer, and work on a JSON implementation of that interface.
-
Donal Fellows over 11 yearsLooks like a very simple SOAP object (there's a
@WebService
annotation on the class) with defaults throughout for how to do the SOAP mapping.