Convert std::ostream to std::string
Solution 1
If you have a a
stringstream
or aostreamstring
, object or reference, just use theostringstream::str()
method that does exactly what you want: extract astring
. But you know that and you apparently have aostream
object, not astringstream
nor aostreamstring
.If your ostream reference is actually a
ostringstream
or astringstream
, useostream::rdbuf
to get astreambuf
, then use this post to see how to extract astring
.
Example:
#include <iostream>
#include <sstream>
std::string toString( std::ostream& str )
{
std::ostringstream ss;
ss << str.rdbuf();
return ss.str();
}
int main() {
std::stringstream str;
str << "Hello";
std::string converted = toString( str );
std::cout << converted;
return 0;
}
Live demo: http://cpp.sh/6z6c
- Finally, as commented by M.M, if your
ostream
is aofstream
or aniostream
, most likely it clears its buffer so you may not be able to extract the full content as proposed above.
Solution 2
Try this
std::ostringstream stream;
stream << "Some Text";
std::string str = stream.str();
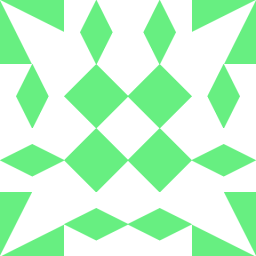
Danny
Updated on June 04, 2022Comments
-
Danny 12 months
Legacy code I maintain collects text into an
std::ostream
. I'd like to convert this to astd::string
.I've found examples of converting
std::sstringstream
,std::ostringstream
etc tostd::string
but not explicitlystd::ostream
tostd::string
.How can I do that? (Ancient C++ 98 only, no boost please)
-
M.M about 6 yearsI don't think this works; the post you link to refers to an input stream's
rdbuf
. -
jpo38 about 6 years@M.M: Just tested that (see live demo added to my post), it works when
ostream
is actually astringstream
, so I d'ont see why it would not work with others (likeofstream
). -
M.M about 6 yearsstringstreams always hold the content in memory, whereas ofstreams don't, I'd expect they clear their buffer once written the current line or whatever
-
jpo38 about 6 yearsBy the way, OP says
I've found examples of converting std::ostringstream to std::string
, so he knows that... -
jpo38 about 6 years@M.M: You are probably right, I commented my post to mention that. Maybe the answer won't work when
ostream
is not actually aostringstream
...