Convert String to Int. String value = scanner input.....hard to explain
49,377
Solution 1
You should use an argument in parseInt
:
int num2 = Integer.parseInt(num1);
and
if (num2 >= 275)
...
Solution 2
Try this:
try{
num2 = Integer.parseInt(num1);
}
catch(Exception ex) {
System.out.println("Something went wrong, the string could not be converted to int.");
}
the try-catch is pretty important because the string could contain characters that cannot convert to int, ofc you need to catch it better than this, but keep it in mind
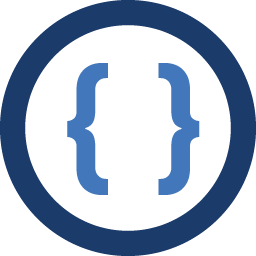
Author by
Admin
Updated on July 19, 2020Comments
-
Admin almost 4 years
Okay so i'm trying to convert a couple things.
So i have already converted my Scanner to a String, now what I want to do is, take the value that they input and use it as an integer for a couple else if statements. IT WONT WORK!
Here is my code so far:
import java.util.Scanner; public class apples { public static void main(String args[]) { Scanner fname = new Scanner(System.in); Scanner sname = new Scanner(System.in); Scanner number = new Scanner(System.in); tuna weight = new tuna(); System.out.println("Enter Your First Name: "); String fname1 = fname.nextLine(); String fnames = fname1; System.out.println("Enter Your Last Name: "); String sname1 = sname.nextLine(); String snames = sname1; System.out.println("Enter Your Weight (Lbs.) : "); String num = number.nextLine(); String num1 = num; System.out.println("Okay " + fname1 + " " + sname1 + " I can see here that you weigh " + num + "lbs."); int num2 = num1.parseInt(); if (num1 >= 275) System.out .println("You know, you should maybe consider laying off the candy my friend....."); }
}
-
Admin over 11 yearsThanks a ton man! I was using the parseInt but didnt know about the (num1); part. Thanks again!
-
MadProgrammer over 11 years+1 for the exception handling (although I'd prefer a ParseException ;))
-
MarsAtomic over 8 yearsWhat's the purpose of answering a question that was both asked and satisfactorily answered three years ago? And to top it off, you didn't even bother to explain the changes you made that caused you to consider it "up a notch."