convert timestamp into current date in android
Solution 1
private String getDate(long time) {
Calendar cal = Calendar.getInstance(Locale.ENGLISH);
cal.setTimeInMillis(time * 1000);
String date = DateFormat.format("dd-MM-yyyy", cal).toString();
return date;
}
notice that I put the time in setTimeInMillis as long and not as int, notice my date format has MM and not mm (mm is for minutes, and not months, this is why you have a value of "41" where the months should be)
for Kotlin users:
fun getDate(timestamp: Long) :String {
val calendar = Calendar.getInstance(Locale.ENGLISH)
calendar.timeInMillis = timestamp * 1000L
val date = DateFormat.format("dd-MM-yyyy",calendar).toString()
return date
}
COMMENT TO NOT BE REMOVED: Dear Person who tries to edit this post - completely changing the content of the answer is, I believe, against the conduct rules of this site. Please refrain from doing so in the future. -LenaBru
Solution 2
For converting time stamp to current time
Calendar calendar = Calendar.getInstance();
TimeZone tz = TimeZone.getDefault();
calendar.add(Calendar.MILLISECOND, tz.getOffset(calendar.getTimeInMillis()));
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss", Locale.getDefault());
java.util.Date currenTimeZone=new java.util.Date((long)1379487711*1000);
Toast.makeText(TimeStampChkActivity.this, sdf.format(currenTimeZone), Toast.LENGTH_SHORT).show();
Solution 3
convert timestamp into current date:
private Date getDate(long time) {
Calendar cal = Calendar.getInstance();
TimeZone tz = cal.getTimeZone();//get your local time zone.
SimpleDateFormat sdf = new SimpleDateFormat("dd/MM/yyyy hh:mm a");
sdf.setTimeZone(tz);//set time zone.
String localTime = sdf.format(new Date(time) * 1000));
Date date = new Date();
try {
date = sdf.parse(localTime);//get local date
} catch (ParseException e) {
e.printStackTrace();
}
return date;
}
Solution 4
DateFormat df = new SimpleDateFormat("HH:mm", Locale.US);
final String time_chat_s = df.format(time_stamp_value);
The time_stamp_value variable type is long
With your code it will look something like so:
private String getDate(long time_stamp_server) {
SimpleDateFormat formatter = new SimpleDateFormat("dd-mm-yyyy");
return formatter.format(time_stamp_server);
}
I change "milliseconds" to time_stamp_server. Consider changing the name of millisecond to "c" or to something more global. "c" is really good because it relates to time and to computing in much more global way than milliseconds does. So, you do not necessarily need a calendar object to convert and it should be as simple as that.
Solution 5
If your result always returns 1970, try this way :
Calendar cal = Calendar.getInstance(Locale.ENGLISH);
cal.setTimeInMillis(timestamp * 1000L);
String date = DateFormat.format("dd-MM-yyyy hh:mm:ss", cal).toString();
You need to multiple your TS value by 1000
It’s so easy to use it.
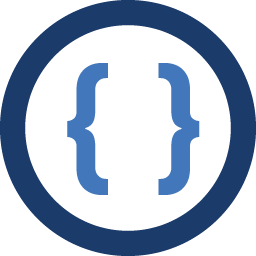
Admin
Updated on January 05, 2021Comments
-
Admin over 3 years
I have a problem in displaying the date,I am getting timestamp as 1379487711 but as per this the actual time is 9/18/2013 12:31:51 PM but it displays the time as 17-41-1970. How to show it as current time.
for displaying time I have used the following method:
private String getDate(long milliSeconds) { // Create a DateFormatter object for displaying date in specified // format. SimpleDateFormat formatter = new SimpleDateFormat("dd-mm-yyyy"); // Create a calendar object that will convert the date and time value in // milliseconds to date. Calendar calendar = Calendar.getInstance(); calendar.setTimeInMillis((int) milliSeconds); return formatter.format(calendar.getTime()); }